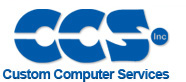 |
 |
View previous topic :: View next topic |
Author |
Message |
Shardik Guest
|
Compare data |
Posted: Thu Apr 26, 2007 10:30 am |
|
|
I need to compare two ip address, this is my code:
typedef struct {
int v[4];
} ip_addr;
And the problem:
ip_addr ip1=192,168,6,5;
ip_addr ip2=192,168,6,5;
How can i compare this two ip?
ip1==ip2 doesnt work, and ip must be a ip_addr type, what can i do? with pointers? |
|
 |
frequentguest Guest
|
|
Posted: Thu Apr 26, 2007 10:59 am |
|
|
A good way to deal with IP addresses is to use a union.
Code: | typedef union
{
int8 bytes[4];
int32 ip
} ip_addr; |
This way you can easily deal with the IP address byte-wise or as a whole.
Code: | ip_addr ip1;
ip1.bytes[0]=255;
ip1.ip=0xFFFFFFFF;
| [/code] |
|
 |
treitmey
Joined: 23 Jan 2004 Posts: 1094 Location: Appleton,WI USA
|
|
Posted: Thu Apr 26, 2007 11:04 am |
|
|
yup Code: | #include <16F877.h>
#use delay(clock=4000000)
#fuses HS,NOWDT,NOLVP
#use rs232(baud=19200,xmit=PIN_C0,invert,stream=DEBUG,disable_ints) // stderr(same as debug)
#use fast_io (a)
union ip_def
{
int8 oct[4];
int32 combined;
};
union ip_def ip_i;
union ip_def ip_o;
//======================= Main ==============================
void main(void)
{
ip_i.oct[0]=255;
ip_i.oct[1]=255;
ip_i.oct[2]=255;
ip_i.oct[3]=255;
ip_o.oct[0]=255;
ip_o.oct[1]=255;
ip_o.oct[2]=255;
ip_o.oct[3]=1;
tris_a=0xFF;
setup_adc_ports(NO_ANALOGS);
setup_adc(ADC_OFF);
if(ip_i.combined != ip_o.combine) fprintf(DEBUG,"nope\n\r");
while(1)
{
}
}
|
|
|
 |
Shardik Guest
|
Thx |
Posted: Thu Apr 26, 2007 3:41 pm |
|
|
Thxs a lot for the replys, it really help me, but im still having some problems:
Code: |
typedef union{
int8 v[4];
int32 combined;
} ip_addr;
typedef struct {
mac_addr mac;
ip_addr ip;
} arp_table;
arp_table tabla;
ip_addr sr;
tabla.ip=192,168,8,3;
sr=192,168,8,3;
if(tabla.ip.combined==sr.combined) printf(lcd_putc,"YES");
else printf(lcd_putc,"NO");
|
Nevers says YES!!! Do you know where is the problem? |
|
 |
Shardik Guest
|
|
Posted: Thu Apr 26, 2007 3:48 pm |
|
|
And how can i do with mac address? (6byte addres)
union ip{
int8 v[4];
int32 combined;
}
union mac{
int8 v[6]:
??? combined;
} |
|
 |
frequentguest Guest
|
|
Posted: Thu Apr 26, 2007 3:58 pm |
|
|
The purpose of the union is that you can treat the ip address as either a 32-bit int or as 4 individual bytes. I imagine the compiler doesn't know what to do with these lines:
Quote: | Code: | tabla.ip=192,168,8,3;
sr=192,168,8,3; | |
you can use: Code: |
tabla.ip.v[0]=192;
tabla.ip.v[1]=168;
tabla.ip.v[2]=8;
tabla.ip.v[3]=3;
//or easier yet
tabla.ip.combined=0xC0A80803; |
and then do your comparison |
|
 |
frequentguest Guest
|
|
Posted: Thu Apr 26, 2007 3:59 pm |
|
|
as for the mac address issue, since the compiler can't handle a 48-bit variable, look at the memcpy() function of the compiler. |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|