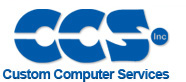 |
 |
View previous topic :: View next topic |
Author |
Message |
Neutone
Joined: 08 Sep 2003 Posts: 839 Location: Houston
|
Flashing LED's beyond on and off |
Posted: Fri Dec 08, 2006 3:29 pm |
|
|
It's probably one of the first things most people learn to do with code on an embedded processor. A lot of circuits don't have much of a way to display information other than a single LED. A single LED can have 5 completely distinct visual states.
Off
Flash 1/2 second on/off
Strobe 1/8 second on/off
Flash/Strobe
On
I like to have the LED off only when the circuit has no power. That means I only need to control 4 states when power is on. I usually have 2 bits that carry some kind of information. Assume you want to show when an alarm occurs and when some process is happening.
Code: |
Int16 LED_period=0;
Int1 LED_Alarm=0;
Int1 LED_Process=0;
Void Service_LED(Void)
{ if(LED_period)
{ LED_period--;
}
Else
{ LED_period=4000;
}
Status_LED=(bit_test(LED_period,8) || !LED_Alarm)
&& (bit_test(LED_period,6) || !LED_Process);
} |
The service routine needs to be called every mS as written. The rate can be changed by changing the bits tested in the bit_test. The alarm and process bits are modified elsewhere. This compiles to a very small size and has very low execution time as well. This can be reduced if you already have a mSecond variable that can be used in the place of LED_period. |
|
 |
Storic
Joined: 03 Dec 2005 Posts: 182 Location: Australia SA
|
|
Posted: Sat Dec 09, 2006 4:07 pm |
|
|
With the Status_LED,I am not sure of the bit
is it a variable or the output bit (led)
below I assigned Status_LED as a bit 2 of Port B
Code: | #byte State_L = 0xF81 // PORT B; PORT A = 0xF80
#bit Status_LED = State_L.2
|
my question is How did you assigned Status_LED, I will get to try the above code within the next few weeks.
Andrew _________________ What has been learnt if you make the same mistake?  |
|
 |
Neutone
Joined: 08 Sep 2003 Posts: 839 Location: Houston
|
|
Posted: Sun Dec 10, 2006 12:51 am |
|
|
Did you set the pin as an output?
This example code flashes an LED that lights up when the output on pin B5 goes low. I ran this on the CCS developers kit for PIC18F452.
Code: | #include <18F452.h>
#fuses NOWDT,WDT128,HS, NOOSCSEN, BROWNOUT, BORV20, PUT, STVREN, NOLVP
#use delay(clock=20000000)
#byte Port_B = 0xF8A
#bit Status_LED = Port_B.5
Int16 mS_Counter;
Int16 Second_Counter;
Int1 LED_Alarm;
Int1 LED_Process;
Void Service_LED(Void)
{ Status_LED=!((bit_test(mS_Counter,8) || !LED_Alarm)
&& (bit_test(mS_Counter,5) || !LED_Process));
}
#int_TIMER2
TIMER2_isr()
{ mS_Counter++;
if(mS_Counter>999)
{ mS_Counter=0;
Second_Counter++;
}
}
void main()
{ setup_timer_2(T2_DIV_BY_4,249,5);
enable_interrupts(INT_TIMER2);
enable_interrupts(GLOBAL);
SET_TRIS_B(0b11001111);
while(1)
{ Service_LED();
LED_Alarm=bit_test(Second_Counter,3); // For lack of a real alarm just turn on and off
LED_Process=bit_test(Second_Counter,4); // For lack of a real process just turn on and off
}
} |
|
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|