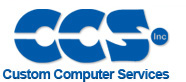 |
 |
View previous topic :: View next topic |
Author |
Message |
Richter
Joined: 18 Mar 2005 Posts: 8
|
Chained List, structs, pointer to pointer and mystery output |
Posted: Wed May 04, 2005 1:27 am |
|
|
Hi there again I`, despairing on this issue:
I`m using a PIC18F6720 the CCS C Compiler Vers:3.180
I try to program a dynamic Messagequeue. I have tried a lot but the output in different levels are ALL different. Even the Operator = seems not to do the rigth thing... here is my Code:
Code: |
#include <18F6720.h>
#DEVICE ICD=TRUE // delete line #device ICD=TRUE to get a standalone application + see fuses !!
#DEVICE ADC=10
#pragma USE DYNAMIC_MEMORY // For using malloc or any other dynamic memory functions.
#include "pins.h"
#use delay(clock=20000000) // oscilator = 20 Mhz
#use rs232 (baud=115200, xmit=Pin_TX1, rcv=Pin_RX1, STREAM=COM2) // external comunication
#use rs232 (baud=115200, xmit=Pin_TX2, rcv=Pin_RX2, STREAM=COM1) // internal comunication Pic2Pic
#fuses HS,NOWDT,WDT1,STVREN,DEBUG //Fuses: HS = High speed osc( > 4mhz), NOWDT = No watchdog timer, WDT1 = WDT uses 1:1 Postscale
//!! NODEBUG should be inserted for standalone application !!
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdlibm.h>
#include"defines.h" // only useful fpr type and status
#include <limits.h>
struct message_Handler
{
int type;
int value;
int status;
char* text[256];
struct message_Handler* next;
};
typedef struct message_Handler message;
int insert_Message( message *given_One);
message *root[1];
main()
{
char input;
char* text[256] = "\0";
int i;
message handler;
fprintf( COM2, " CASE1\r\n");
handler.type = REPORT; //2
handler.status = ACTIVE; //1
handler.value = 6;
insert_Message( &handler );
fprintf( COM2, "\n\r\n Value:%d\r\n type:%d\r\n status:%d", (root[0])->value, (root[0])->type, (root[0])->status);
int insert_Message( message* given_One)
{
message *anew, *seq, *pre;
// new Message will created:
anew = ( message* ) malloc( sizeof( message ) );
// I think here something wrong!!!
anew->value = given_One->value;
anew->type = given_One->type;
anew->status = given_One->status;
fprintf( COM2, "\n\rinsert function: given_One\r\n Wert:%d\r\n type:%d\r\n status:%d", given_One->value, given_One->type, given_One->status);
fprintf( COM2, "\n\rinsert function: new\r\n Wert:%d\r\n type:%d\r\n status:%d", anew->value, anew->type, anew->status);
if( 256 > strlen( given_One->text ) )
{
strcpy( anew->text, given_One->text );
}
else
{
return FALSE;
}
if( root[0] == NULL || (root[0])->status == OBSOLETE )
{ // insert @ begining:
anew->next = *root;
root[0] = anew;
return TRUE;
}
else
{ // insert after last ACTIVE
pre = *root;
seq = (root[0])->next;
// searching pos.
while( seq != NULL && seq->status != OBSOLETE )
{
pre = pre->next;
seq = seq->next;
}
// inserting...
anew->next = seq;
pre->next = anew;
return TRUE;
}
}
|
Note that every fprintf puts a different Value!!!!!!!! Am I doing starting failueres ? PLZ Help me it drives me crazy  _________________ Why I get all these strange problems ? |
|
 |
Richter
Joined: 18 Mar 2005 Posts: 8
|
|
Posted: Wed May 04, 2005 2:51 am |
|
|
OK I found my error:
malloc returns NULL!!!!!
so he didn t allocated memory anytime!!!!!
But now I have a nother problem: How can I force my PIC to allocate memory???
I got another question:
what exactly does #pragma use dynamic memory ??
When I delete this line my compiler finds a failure in the memorymanagementunit!!!!! Damn!
Can anybody help me on this issue?  _________________ Why I get all these strange problems ? |
|
 |
ckielstra
Joined: 18 Mar 2004 Posts: 3680 Location: The Netherlands
|
|
Posted: Wed May 04, 2005 3:49 am |
|
|
I never used malloc in CCS so can't help you on this.
In fact I avoid all malloc's in embedded programs! The problem with malloc/free is that in the long term you'll end up with fragmented memory and can't allocate a large memory block anymore. To solve this a reset is unacceptable and a memory cleanup is bad for real-time requirements.
Often when taking a second look at your requirements you'll see you can do without the malloc. For example, you know the total amount of memory available so you can allocate the maximum possible array of structures at compile time. Doing so on a PC platform would have you killed by the end-user, but in embedded it is the preferred way.
Another bug in your code (existing twice in your code):This allocates an array of pointers to characters, but you are using it as a pointer to a character array. Change to: |
|
 |
Ttelmah Guest
|
|
Posted: Wed May 04, 2005 3:50 am |
|
|
Key thing is that malloc, can only return a block of memory up to 256 bytes in length. If you look at the manual entry for the call, it says that the 'size' is an integer.
You could get 'close' to what you want, by redefining the structure, so that instead of holding a 256byte character array, it holds a pointer to a 255 byte array. Allocate space for the now much smaller structure, then use malloc again to allocate storage for this, and put the returned pointer into the structure.
Realistically, you are probably going to have to start thinking about doing some 'DIY' memory management. If you reserve a block covering the top few pages of the RAM, from use by the compiler, you can write a fairly basic allocate/de-allocate handler to use this. In the past, I had to do this on a somewhat smaller chip...
Best Wishes |
|
 |
Richter
Joined: 18 Mar 2005 Posts: 8
|
|
Posted: Wed May 04, 2005 4:10 am |
|
|
THNX GUYS, U Are the BEST
@ckielstra: Yeah you are right! thnx. The last error was an error caused by my hushy program style (sorry, and thnx)
I need this malloc because I want to program a dynamic Messagequeue I don t know when and through what the messages will come! Is there another option???
@Ttelmah: That was a damn good idea! thnx! But actually I think I will reduce the number of chars in the Message text!
THNX again! I m so happy that u answered me so quickly!  _________________ Why I get all these strange problems ? |
|
 |
tatiq Guest
|
Re: Chained List, structs, pointer to pointer and mystery ou |
Posted: Mon Sep 19, 2005 6:29 am |
|
|
hello,
i need the ccs PCM C Compiler version 3.180,
where can i find it?
where can i find a demo-version.
[email protected]
thx in advence. |
|
 |
Ttelmah Guest
|
|
Posted: Mon Sep 19, 2005 7:18 am |
|
|
You won't.
You might find an old version on a software companies shelf somewhere, but the oldest version currently supplied is 3.191. Why on earth would you want a version this old?.
There is a limited downloadable 'demo' version of the CCS, which only supports the 16F877, 16C544, and 18F458, with a 2KB program size limit.
CCS, might be able to supply an older version, if it is needed because of some program change which stops the code working with a latter version. You would need to talk to them about this.
Best Wishes |
|
 |
Tariq Guest
|
|
Posted: Mon Sep 19, 2005 2:33 pm |
|
|
Where can i find the old version.
i downloaded the demo-version from the link :
http://www.ccsinfo.com/demo.shtml
but that don't do for my programm
thx |
|
 |
Ttelmah Guest
|
|
Posted: Mon Sep 19, 2005 2:48 pm |
|
|
If the demo won't do what you want, then the only solution is to talk to CCS, and see if they can supply you the older compiler if you buy the current version. Even though this is an 'old' compiler, it is still a purchasable item, not something available for free download.
Best Wishes |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
 |
Tariq Guest
|
|
Posted: Mon Sep 19, 2005 3:34 pm |
|
|
How much costs a later version .
exist a student version?
have i the possibility to translate the C-code in assemblers or cc5x code?
Thank you in advance. |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
 |
Mark
Joined: 07 Sep 2003 Posts: 2838 Location: Atlanta, GA
|
|
Posted: Mon Sep 19, 2005 5:27 pm |
|
|
For $125 you can't go wrong. |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|