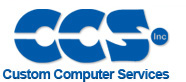 |
 |
View previous topic :: View next topic |
Author |
Message |
JEliecer
Joined: 08 Feb 2005 Posts: 17 Location: Bucaramanga - Colombia
|
Routines for LCD |
Posted: Tue Feb 08, 2005 6:05 pm |
|
|
Hi,
I need a routine to manage a lcd (2x16) , with a pic 18f448.
I need help, since I am new programming.
Thank you.
Until soon. |
|
 |
dyeatman
Joined: 06 Sep 2003 Posts: 1933 Location: Norman, OK
|
|
Posted: Tue Feb 08, 2005 9:12 pm |
|
|
There is lots of info on working with an LCD on this forum. Try a little searching on LCD in this forum before you start asking for someone to do the work for you...
You wont find anything for an 18F448 specifically but its basically all the same... |
|
 |
JEliecer
Joined: 08 Feb 2005 Posts: 17 Location: Bucaramanga - Colombia
|
I have a routine for lcd with the 18F448 |
Posted: Fri Feb 25, 2005 9:15 am |
|
|
//////////////////////////////////////////////////////////////////////////
// LCDPIC18.C //
// DRIVER FOR LCD //
// //
// lcd_init() Debe ser llamada antes que las otras funciones. //
// //
// lcd_putc(c) Visualiza c en la siguiente posici�n del display. //
// Caracteres especiales de control: //
// \f Borrar display //
// \n Saltar a la segunda linea //
// \b Retroceder una posici�n. //
// //
// lcd_gotoxy(x,y) Nueva posici�n de escritura en el display. //
// (la esquina superior izquierda es 1,1) //
// //
// lcd_getc(x,y) Devuelve el caracter de la posici�n x,y //
// //
// LCD (pin) PIC (pin) //
// 1 GND ----- //
// 2 VCC ----- //
// 3 Contrast ----- //
// 4 RS RA0 //
// 5 RW RA1 //
// 6 ENABLE RA2 //
// 7 D0 RB0 //
// . . . //
// . . . //
// . . . //
// 14 D7 RB7 //
// Si existen: //
// 15 VCC LIGTH //
// 16 GND LIGTH //
// //
//////////////////////////////////////////////////////////////////////////
#bit lcd_enable = 0xF80.2
#bit lcd_rw = 0xF80.1
#bit lcd_rs = 0xF80.0
#byte lcd_a = 0xF80
#byte lcd_b = 0xF81
#define LCD_LINEA2 0x40 // Direcci�n de memoria para la segunda l�nea
#define LCD_DATO 1
#define LCD_COMANDO 0
#define LCD_CLEAR 0x01 //Borra el LCD
#define LCD_HOME 0x02 //Env�a el cursor a la posici�n (1,1)
#define LCD_DISPLAY_OFF 0x08 //Apaga el LCD
#define LCD_DISPLAY_ON 0x0C //Enciende el LCD
#define LCD_CURSOR_ON 0x0E //Visualiza el Cursor
#define LCD_CURSOR_BLINK 0x0F //El cursor [spam]�la
#define LCD_CURSOR_SHIFT_RIGHT 0x10 //Para posicionar el cursor
#define LCD_CURSOR_SHIFT_LEFT 0x14 //Para posicionar el cursor
#define LCD_DISPLAY_SHIFT_RIGHT 0x18 //Para posicionar el cursor
#define LCD_DISPLAY_SHIFT_LEFT 0x1C //Para posicionar el cursor
//Rutina para leer los datos del LCD
int lcd_leer()
{
int valor;
set_tris_a(0x18);
set_tris_b(0xFF);
lcd_rw = 1;
delay_cycles(1);
lcd_enable = 1;
delay_cycles(1);
valor = lcd_b;
lcd_enable = 0;
delay_cycles(1);
set_tris_b(0x00);
return valor;
}
//Funci�n para enviar datos al LCD
void lcd_enviar(int dir, int valor)
{
set_tris_a(0x00);
set_tris_b(0x00);
lcd_rs = 0;
while( bit_test(lcd_leer(),7) );
lcd_rs = dir;
delay_cycles(1);
lcd_rw = 0;
delay_cycles(1);
lcd_enable = 0;
lcd_b = valor;
delay_cycles(1);
lcd_enable = 1;
delay_us(2);
lcd_enable = 0;
}
//RUTINA DE INICIO DEL LCD
void lcd_init()
{
int i;
set_tris_a(0x18);
set_tris_b(0x00);
lcd_enable = 0;
lcd_rw = 0;
lcd_rs = 0;
delay_ms(15);
for(i=0; i<3; i++)
{
lcd_enviar(LCD_COMANDO,0x38);
delay_ms(5);
}
lcd_enviar(LCD_COMANDO,LCD_DISPLAY_ON);
lcd_enviar(LCD_COMANDO,0x06);
lcd_enviar(LCD_COMANDO,LCD_CLEAR);
lcd_enviar(LCD_COMANDO,LCD_HOME);
}
//Rutina para enviar datos a una posicion especifica en el LCD
void lcd_gotoxy( byte x, byte y)
{
byte dir;
if(y!=1)
dir=LCD_LINEA2;
else
dir=0;
dir+=x-1;
lcd_enviar(LCD_COMANDO,0x80|dir);
}
//Rutina para enviar caracteres al LCD
void lcd_putc( char c)
{
switch (c)
{
case '\f' : lcd_enviar(LCD_COMANDO,0x01);
delay_ms(2);
break;
case '\n' : lcd_gotoxy(1,2);
break;
case '\b' : lcd_enviar(LCD_COMANDO,0x10);
break;
default : lcd_enviar(LCD_DATO,c);
break;
}
}
char lcd_getc( int x, int y)
{
char valor;
lcd_gotoxy(x,y);
lcd_rs = 1;
valor = lcd_leer();
lcd_rs = 0;
return valor;
}
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
Este es el programa para sacar datos por el LCD. Esta listo para probar. Solo tienes que armar el Hardware, y ya funciona.
////////////////////////////////////////////////////////////////////////////
//// LCD448.C ////
//// ////
//// Este programa lee y escribe en un LCD ////
//// Utilizando la libreria LCDPIC16.C ////
////////////////////////////////////////////////////////////////////////////
#include <18f448.h>
#use delay(clock=20000000,RESTART_WDT)
#include <lcdpic18.c>
#use fast_io(A)
#use fast_io(B)
//#byte PORTA = 0xf80
//#byte PORTB = 0xf81
//#byte PORTC = 0xf82
void main(void)
{
lcd_init(); // Comandos de inicializaci�n del LCD.
while(1)
{
restart_wdt();
lcd_enviar(LCD_COMANDO, LCD_CLEAR);
delay_ms(2);
lcd_enviar(LCD_COMANDO, LCD_HOME);
delay_ms(2);
lcd_putc(" SoY Un MaLdItO GeNiO\n"); // con \n salta a la segunda l�nea
lcd_putc("...Si O No InIs JoSE...");
delay_ms(4000);
restart_wdt();
lcd_enviar(LCD_COMANDO, LCD_CLEAR);
delay_ms(2);
lcd_enviar(LCD_COMANDO, LCD_HOME);
delay_ms(2);
lcd_putc(" PoNgAsE A TrAbAjAr\n");
//lcd_gotoxy(5,2); // Otra forma de saltar a la segunda l�nea
lcd_putc(" PoRqUe AuN No Ha HeChO");
delay_ms(4000);
restart_wdt();
lcd_enviar(LCD_COMANDO, LCD_CLEAR);
delay_ms(2);
lcd_enviar(LCD_COMANDO, LCD_HOME);
delay_ms(2);
lcd_putc(" Ni UnA PuTa MiErDa Y...\n");
lcd_putc("...EsToy QuE Lo MaTo...");
delay_ms(4000);
restart_wdt();
lcd_enviar(LCD_COMANDO, LCD_CLEAR);
delay_ms(2);
lcd_enviar(LCD_COMANDO, LCD_HOME);
delay_ms(2);
lcd_putc(" PaRa QuE ApReNdA a SeR\n");
lcd_putc(" RESPONSABLE");
delay_ms(4000);
}
}
Para preguntas contactame a [email protected] |
|
 |
Humberto
Joined: 08 Sep 2003 Posts: 1215 Location: Buenos Aires, La Reina del Plata
|
|
Posted: Fri Feb 25, 2005 9:44 am |
|
|
JEliecer wrote:
Quote: |
lcd_putc(" Ni UnA PuTa MiErDa Y...\n");
lcd_putc("...EsToy QuE Lo MaTo...");
|
With this kind of messages in LCD I can�t imagine what is your project...
Humberto |
|
 |
JEliecer
Joined: 08 Feb 2005 Posts: 17 Location: Bucaramanga - Colombia
|
|
Posted: Mon Feb 28, 2005 1:18 pm |
|
|
Humberto wrote: | JEliecer wrote:
Quote: |
lcd_putc(" Ni UnA PuTa MiErDa Y...\n");
lcd_putc("...EsToy QuE Lo MaTo...");
|
With this kind of messages in LCD I can�t imagine what is your project...
Humberto |
This lines are a JOKE for a friend.
You can replace it for the text that you need.
I hope you don't have but inconveniences.
Until soon.
P.D: Please, write me in Spanish. |
|
 |
Humberto
Joined: 08 Sep 2003 Posts: 1215 Location: Buenos Aires, La Reina del Plata
|
|
Posted: Mon Feb 28, 2005 2:32 pm |
|
|
Hola JEliecer,
Of course that I don�t have any inconvenience with the text !!!
My comments was just for curiosity.
If you want I can write you in Spanish as a private message,
not here in the forum.
Saludos,
Humberto |
|
 |
Mark
Joined: 07 Sep 2003 Posts: 2838 Location: Atlanta, GA
|
|
Posted: Mon Feb 28, 2005 9:41 pm |
|
|
"SoY Un MaLdItO GeNiO"
You'd think being a Genius he wouldn't be posting  |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|