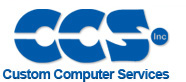 |
 |
View previous topic :: View next topic |
Author |
Message |
Spiffy
Joined: 21 Jan 2004 Posts: 12
|
Sampling an 8 bit WAV file with a PIC |
Posted: Tue Feb 10, 2004 12:44 pm |
|
|
Hey! spam -- ignore this post to all the interestee`!
How would I sample a 8 bit audio WAV file through my PIC and have good sound fidelity?
heres what I cam up with:
#include "16f877.h"
#include "stdlib.h"
#use delay (clock=4000000)
#use rs232 (baud=9600,xmit=pin_c6,rcv=pin_c7)
#bit CKE = 0x94.6
#bit SMP = 0x94.7
#bit CKP = 0x14.4
#byte SSPSTAT=0x94
#byte SSPCON=0x14
#bit ADFM=0x9f.7
//D�claration des variables
char choix;
int data=0,yx,res2;
long int i=0,resultat;
void son (void);
void ecriture (void);
void lecture (void);
void conversion (void);
void main (void)
{
set_tris_C(0x90); //configure les ports pour sortie et entree.
set_tris_A(0xff);
set_tris_E(0x04);
setup_adc_ports(ALL_ANALOG);
setup_adc(ADC_CLOCK_internal);
ADFM=1;
setup_spi(spi_master|spi_l_to_h|spi_clk_div_4);
CKE = 1; // donn�e VALIDE de front descendant � front descendant
CKP = 0; // La m�moire �chantillone sur front montant
SMP = 0; // �chantillonage au centre d'un cr�neau
while(1)
{
i=0;
Printf("\n\r 1-Pour faire une simple ecriture");
Printf("\n\r 2-Pour faire une lecture");
Printf("\n\r 3-Pour faire une conversion AD");
Printf("\n\r 4-Pour faire jouer un son");
choix=getch();
if (choix=='1')
{
ecriture();
}
if (choix=='2')
{
lecture();
}
if (choix=='3')
{
conversion();
}
if (choix=='4')
{
son();
}
else
{
puts("\n\r Erreur");
}
}
}
void ecriture (void)
{
printf("\n\r appuyer sur UN chiffre:");
yx=getch();
printf("\n\rlecture:%d",yx);
getch();
output_low(pin_a4); //On choisi la memoire
spi_write(0x06); //Write enable
output_high(pin_a4);
output_low(pin_a4);
spi_write(0x02); //on veut ecrire sur la memoire
spi_write(0x00); //On choisi l'adress 1
spi_write(0x00); //On choisi l'adress 2
While(i<70)
{
spi_write(yx); //On designe le data a envoyer
i++;
}
output_high(pin_a4); //On enleve la selection de memoire
printf("\n\r ok!");
}
void lecture (void)
{
printf("\n\r SSPSTAT:%x",SSPSTAT);
printf("\n\r SSPSTAT:%x",SSPCON);
printf("\n\rReading...");
output_low(pin_a4);
spi_write(0x03);
spi_write(0x00);
spi_write(0x00);
While(i<80)
{
data=spi_read(0);
printf("\n\rlecture: %lu %x",i,data);
i++;
}
output_high(pin_a4);
}
void conversion (void)
{
set_adc_channel(2);
output_low(pin_a4); //On choisi la memoire
spi_write(0x06); //Write enable
output_high(pin_a4);
output_low(pin_a4);
spi_write(0x02); //on veut ecrire sur la memoire
spi_write(0x00); //On choisi l'adress 1
spi_write(0x00); //On choisi l'adress 2
While(i<60)
{
resultat=read_adc();
res2=(int)(resultat/4);
spi_write(res2); //On designe le data a envoyer
i++;
puts("valeur entree");
}
output_high(pin_a4);
}
void son (void)
{
output_low(pin_a5);
output_low(pin_a4);
spi_write(0x03);
spi_write(0x00);
spi_write(0x00);
While(i<80)
{
data=spi_read(0);
printf("\n\rlecture: %lu %x",i,data);
i++;
}
output_high(pin_a4);
output_high(pin_a5);
}
The main idea here is I want to sample the sound and and write to a EEPROM memory so I could reuse this sound later on and convert it with a CDA converter.
And also which CDA ( digital to analog convertor) is suitable for this task I have a MAX522 do you any idea if its good or not? |
|
 |
Neutone
Joined: 08 Sep 2003 Posts: 839 Location: Houston
|
|
Posted: Tue Feb 10, 2004 1:08 pm |
|
|
There has been some post on a 1bit audio solution that works very well. |
|
 |
Guest
|
Re: Sampling an 8 bit WAV file with a PIC |
Posted: Thu Dec 08, 2005 7:20 am |
|
|
Spiffy wrote: | Hey! spam -- ignore this post to all the interestee`!
How would I sample a 8 bit audio WAV file through my PIC and have good sound fidelity?
heres what I cam up with:
#include "16f877.h"
#include "stdlib.h"
#use delay (clock=4000000)
#use rs232 (baud=9600,xmit=pin_c6,rcv=pin_c7)
#bit CKE = 0x94.6
#bit SMP = 0x94.7
#bit CKP = 0x14.4
#byte SSPSTAT=0x94
#byte SSPCON=0x14
#bit ADFM=0x9f.7
//D�claration des variables
char choix;
int data=0,yx,res2;
long int i=0,resultat;
void son (void);
void ecriture (void);
void lecture (void);
void conversion (void);
void main (void)
{
set_tris_C(0x90); //configure les ports pour sortie et entree.
set_tris_A(0xff);
set_tris_E(0x04);
setup_adc_ports(ALL_ANALOG);
setup_adc(ADC_CLOCK_internal);
ADFM=1;
setup_spi(spi_master|spi_l_to_h|spi_clk_div_4);
CKE = 1; // donn�e VALIDE de front descendant � front descendant
CKP = 0; // La m�moire �chantillone sur front montant
SMP = 0; // �chantillonage au centre d'un cr�neau
while(1)
{
i=0;
Printf("\n\r 1-Pour faire une simple ecriture");
Printf("\n\r 2-Pour faire une lecture");
Printf("\n\r 3-Pour faire une conversion AD");
Printf("\n\r 4-Pour faire jouer un son");
choix=getch();
if (choix=='1')
{
ecriture();
}
if (choix=='2')
{
lecture();
}
if (choix=='3')
{
conversion();
}
if (choix=='4')
{
son();
}
else
{
puts("\n\r Erreur");
}
}
}
void ecriture (void)
{
printf("\n\r appuyer sur UN chiffre:");
yx=getch();
printf("\n\rlecture:%d",yx);
getch();
output_low(pin_a4); //On choisi la memoire
spi_write(0x06); //Write enable
output_high(pin_a4);
output_low(pin_a4);
spi_write(0x02); //on veut ecrire sur la memoire
spi_write(0x00); //On choisi l'adress 1
spi_write(0x00); //On choisi l'adress 2
While(i<70)
{
spi_write(yx); //On designe le data a envoyer
i++;
}
output_high(pin_a4); //On enleve la selection de memoire
printf("\n\r ok!");
}
void lecture (void)
{
printf("\n\r SSPSTAT:%x",SSPSTAT);
printf("\n\r SSPSTAT:%x",SSPCON);
printf("\n\rReading...");
output_low(pin_a4);
spi_write(0x03);
spi_write(0x00);
spi_write(0x00);
While(i<80)
{
data=spi_read(0);
printf("\n\rlecture: %lu %x",i,data);
i++;
}
output_high(pin_a4);
}
void conversion (void)
{
set_adc_channel(2);
output_low(pin_a4); //On choisi la memoire
spi_write(0x06); //Write enable
output_high(pin_a4);
output_low(pin_a4);
spi_write(0x02); //on veut ecrire sur la memoire
spi_write(0x00); //On choisi l'adress 1
spi_write(0x00); //On choisi l'adress 2
While(i<60)
{
resultat=read_adc();
res2=(int)(resultat/4);
spi_write(res2); //On designe le data a envoyer
i++;
puts("valeur entree");
}
output_high(pin_a4);
}
void son (void)
{
output_low(pin_a5);
output_low(pin_a4);
spi_write(0x03);
spi_write(0x00);
spi_write(0x00);
While(i<80)
{
data=spi_read(0);
printf("\n\rlecture: %lu %x",i,data);
i++;
}
output_high(pin_a4);
output_high(pin_a5);
}
The main idea here is I want to sample the sound and and write to a EEPROM memory so I could reuse this sound later on and convert it with a CDA converter.
And also which CDA ( digital to analog convertor) is suitable for this task I have a MAX522 do you any idea if its good or not? |
|
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|