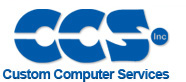 |
 |
View previous topic :: View next topic |
Author |
Message |
Jeff Jackowski Guest
|
Modulo division problem |
Posted: Mon Jul 08, 2002 1:06 pm |
|
|
I'm working on some code that will take a 16-bit unsigned integer with a max value of 34000 and produce a string with the decimal equivalent in ASCII of twice the value in the integer. I have compiled the code with gcc and ran it on my PC. This works as intened, so I know my algorithm is correct.
However, when I compile it with PCM (Linux, v3.091), it only works to convert an 8-bit variable. When given a 16-bit variable, even with a value that will fit within 8-bits, it only outputs the most significant digit. Each following digit is set to the value zero, and not the ASCII '0', but just 0.
Here is the code:
<PRE>#include <stdio.h>
// For PCM, change the following 2 lines to:
// void writeDecVal(char *str, long val) {
// char loop;
void writeDecVal(char *str, int val) {
unsigned char loop;
str[4] = (val \% 5) * 2 + '0';
val /= 5;
for (loop = 3; loop != 0xFF; loop--) {
if (val > 0) {
str[loop] = (val \% 10) + '0';
val /= 10;
}
else {
str[loop] = ' ';
}
}
}
void showit(char *str) {
printf("Result: \%s, \%02X \%02X \%02X \%02X \%02X \%02X\n",
str, str[0], str[1], str[2], str[3], str[4], str[5]);
}
void main() {
char str[10];
int val;
memset(str, 0, 10);
val = 34000;
writeDecVal(str, val);
showit(str);
memset(str, 0, 10);
val = 1;
writeDecVal(str, val);
showit(str);
}
</PRE>
Any idea why this isn't working on the PIC?
___________________________
This message was ported from CCS's old forum
Original Post ID: 5414 |
|
 |
Albert Holman Guest
|
Re: Modulo division problem |
Posted: Thu Jul 18, 2002 3:20 am |
|
|
:=I'm working on some code that will take a 16-bit unsigned integer with a max value of 34000 and produce a string with the decimal equivalent in ASCII of twice the value in the integer. I have compiled the code with gcc and ran it on my PC. This works as intened, so I know my algorithm is correct.
:=
:=However, when I compile it with PCM (Linux, v3.091), it only works to convert an 8-bit variable. When given a 16-bit variable, even with a value that will fit within 8-bits, it only outputs the most significant digit. Each following digit is set to the value zero, and not the ASCII '0', but just 0.
:=
:=Here is the code:
:=
:=<PRE>#include <stdio.h>
:=
:=// For PCM, change the following 2 lines to:
:=// void writeDecVal(char *str, long val) {
:=// char loop;
:=
:=void writeDecVal(char *str, int val) {
:= unsigned char loop;
:=
:= str[4] = (val \% 5) * 2 + '0';
:= val /= 5;
:= for (loop = 3; loop != 0xFF; loop--) {
:= if (val > 0) {
:= str[loop] = (val \% 10) + '0';
:= val /= 10;
:= }
:= else {
:= str[loop] = ' ';
:= }
:= }
:=}
:=
:=void showit(char *str) {
:= printf("Result: \%s, \%02X \%02X \%02X \%02X \%02X \%02X\n",
:= str, str[0], str[1], str[2], str[3], str[4], str[5]);
:=}
:=
:=void main() {
:= char str[10];
:= int val;
:=
:= memset(str, 0, 10);
:= val = 34000;
:= writeDecVal(str, val);
:= showit(str);
:= memset(str, 0, 10);
:= val = 1;
:= writeDecVal(str, val);
:= showit(str);
:=}
:=</PRE>
:=Any idea why this isn't working on the PIC?
Hello Jack,
The problem lies most likely in the size of the int variable.
The size is 8 bit unsigned in the CCS compiler version I use and for other C compilers like you used to test the algorithm, it is probably 16 bit signed or more.
If you change all int declarations to long (size is then 16 bit unsigned) then it should work. At least it worked when I tried it.
good luck
___________________________
This message was ported from CCS's old forum
Original Post ID: 5642 |
|
 |
Al
Joined: 13 Nov 2003 Posts: 28 Location: Belfast
|
Modulo division problem |
Posted: Fri Jan 30, 2004 8:40 am |
|
|
You can actually define a 16 bit integer as int16 using CCS compiler. This should solve your problem.  _________________ Alan Murray |
|
 |
Mark
Joined: 07 Sep 2003 Posts: 2838 Location: Atlanta, GA
|
|
Posted: Fri Jan 30, 2004 9:40 am |
|
|
Dude....that post is over 1.5 years old!!!!!!!!! I don't think he is still waiting. |
|
 |
Al
Joined: 13 Nov 2003 Posts: 28 Location: Belfast
|
|
Posted: Fri Jan 30, 2004 10:08 am |
|
|
Thanks for pointing that out, the processor in my head must have generated an integer division error giving me 0.5 years.  _________________ Alan Murray |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|