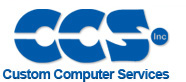 |
 |
View previous topic :: View next topic |
Author |
Message |
art
Joined: 21 May 2015 Posts: 181
|
Convert Binary to Decimal number |
Posted: Wed Mar 06, 2019 12:39 am |
|
|
Hi,
I'm trying to convert 16 digit Binary number to a Decimal number. My code can only convert a 5 digit binary number. If the input is more than 5 digits, it will not convert to decimal number correctly. How to make it convert a 16 digit binary number into a decimal number ? I've converted this code from a C programming example and it can convert 16 binary digits to decimal perfectly.
Code: |
#include <18F4550.h>
#fuses HSPLL,NOWDT,PROTECT,NOLVP,NODEBUG,USBDIV,PLL5,CPUDIV1,VREGEN
#use delay(clock=48000000)
#use rs232(baud=9600, xmit=PIN_C6, rcv=PIN_C7,ERRORS)
#include <string.h>
#include <input.c>
#include <stdio.h>
#include <stdlib.h>
#include <usb_cdc.h>
#include <math.h>
void main()
{
char c;
int8 i ;
char d,key;
long int n1, n,p=1;
long int dec=0,k=1,j,m;
usb_init_cs();
while (TRUE)
{
usb_task();
if (usb_cdc_kbhit())
{
c=usb_cdc_getc();
if (c=='\n') {putc('\r'); putc('\n');}
if (c=='\r') {putc('\r'); putc('\n');}
while(true)
{
d = usb_cdc_getc();
if(d=='B') // push B for Binary conversion
{
while (key!=32) // push SPACE BAR to stop
{
printf(usb_cdc_putc,"Input a binary number :");
&n=get_long_usb();
n1=n;
for (j=n;j>0;j=j/10)
{
m = j % 10;
if(k==1)
p=p*1;
else
p=p*2;
dec=dec+(m*p);
k++;
}
printf(usb_cdc_putc,"\nThe equivalent Decimal Number : %ld \n\n",dec);
} key=0;
}
}
}
}
}
|
|
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19498
|
|
Posted: Wed Mar 06, 2019 1:35 am |
|
|
The problem is using 'get_long_usb'. Long is only a 16bit number. So 0 to
65535. Using this to input a binary number limits you to just 5 digits...
Simple answer is to read the value yourself:
Code: |
char c;
unsigned int32 result;
printf(usb_cdc_putc,"Input a binary number :");
result=0;
while (TRUE)
{
c=usb_cdc_getc();
if (!isamong(c, "01")) //needs 'ctype.h'
break; //not '0' or '1' terminate
result*=2;
result+= c-'0';
}
//'result' now contains the decoded number
printf(usb_cdc_putc,"\nThe equivalent Decimal Number : %ld \n\n",result);
|
For each digit you input simply multiply the result 'so far' by 2, and
add in the value of this digit.
Type anything other than '0' or '1' and it terminates. |
|
 |
art
Joined: 21 May 2015 Posts: 181
|
|
Posted: Wed Mar 06, 2019 2:07 am |
|
|
Dear Ttelmah,
Thank you very much....it works ! |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|