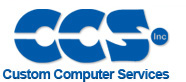 |
 |
View previous topic :: View next topic |
Author |
Message |
runtime
Joined: 15 Sep 2003 Posts: 36
|
Floating Point numbers not supported??? |
Posted: Wed Jan 07, 2004 10:32 am |
|
|
Hello,
I want to do a simple math division and get a result in decimal format, I'm reading a voltage with read_adc() which returns an integer, now I want to divide that number by 2 and get the result with 1 digit after the decimal point, if I try the following I get 'Floating point numbers not supported' from the compiler...
int TRead;
float CValue;
in main:
TRead = read_adc();
CValue = TRead/2.0;
fprintf(COM,"CValue: %3.1f \r\n",CValue);
any ideas?
Thank you in advance |
|
 |
Ttelmah Guest
|
Re: Floating Point numbers not supported??? |
Posted: Wed Jan 07, 2004 10:46 am |
|
|
runtime wrote: | Hello,
I want to do a simple math division and get a result in decimal format, I'm reading a voltage with read_adc() which returns an integer, now I want to divide that number by 2 and get the result with 1 digit after the decimal point, if I try the following I get 'Floating point numbers not supported' from the compiler...
int TRead;
float CValue;
in main:
TRead = read_adc();
CValue = TRead/2.0;
fprintf(COM,"CValue: %3.1f \r\n",CValue);
any ideas?
Thank you in advance |
Which compiler?.
What you show should work (though it is sometimes worth being a bit 'butch' with the compiler, and forcing the type conversion, with code like):
CValue = (float)TRead/2.0;
However your error message, sounds like you might have one of the demo versions that did not have 'float' support.
Best Wishes |
|
 |
Ttelmah Guest
|
Re: Floating Point numbers not supported??? |
Posted: Wed Jan 07, 2004 10:48 am |
|
|
Ttelmah wrote: | runtime wrote: | Hello,
I want to do a simple math division and get a result in decimal format, I'm reading a voltage with read_adc() which returns an integer, now I want to divide that number by 2 and get the result with 1 digit after the decimal point, if I try the following I get 'Floating point numbers not supported' from the compiler...
int TRead;
float CValue;
in main:
TRead = read_adc();
CValue = TRead/2.0;
fprintf(COM,"CValue: %3.1f \r\n",CValue);
any ideas?
Thank you in advance |
Which compiler?.
What you show should work (though it is sometimes worth being a bit 'butch' with the compiler, and forcing the type conversion, with code like):
CValue = (float)TRead/2.0;
However your error message, sounds like you might have one of the demo versions that did not have 'float' support.
Best Wishes |
Worth perhaps adding, that you can get your required result, possibly with less code, and quicker, without using a float, with:
fprintf(COM,"CValue: %2u.%1u \r\n",Tread/2,Tread\2);
Best Wishes |
|
 |
KerryW Guest
|
|
Posted: Wed Jan 07, 2004 4:11 pm |
|
|
or you could do it like this:
int TRead;
in main:
TRead = read_adc();
fprintf(COM,"CValue: %u.%c \r\n",TRead>>2, (TRead&1)?'5':'0');
Which breaks down like this:
fprintf(COM,"CValue:
%u.%c \r\n" // print an unsigned float, a decimal point, a character, and a newe line
,TRead>>2 // print TRead divided by 2 (right shifted once)
, (TRead&1)?'5':'0'); // if TRead is odd, print '5', if it's even, print '0'
The last digit can only be 0 or 5 anyway. This method doesn't use any multiplication or divide routines. It will be very short and very quick. |
|
 |
Alan Murray Guest
|
Floating Point numbers not supported??? |
Posted: Fri Jan 30, 2004 8:18 am |
|
|
The problem is that when you divide 2 decimal numbers the compiler stores the result as a decimal thereby throwing away the remainder.
For dividing by powers of 2 shifting left works as suggested above.
However a much simpler option is to cast both divisor and dividend as floats before doing the division - this will yield a floating point result. e.g.
int adcReading;
float tempADC, result;
tempADC = (float)adcReading;
result = tempADC/2.0;
This will handle all integers - not just powers of 2.  |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|