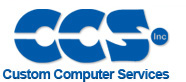 |
 |
View previous topic :: View next topic |
Author |
Message |
art
Joined: 21 May 2015 Posts: 181
|
EX_RTC using USB problem |
Posted: Fri Feb 10, 2017 9:39 pm |
|
|
Hi,
I've modified EX_RTC.c file so it can be use for USB instead of using serial cable.
The problem is, the reply result is always
165/165/165 165:165:165
Need help to solve it
Code: |
#include <18F4550.h>
#fuses HSPLL,NOWDT,PROTECT,NOLVP,NODEBUG,USBDIV,PLL5,CPUDIV1,VREGEN
#use delay(clock=48000000)
#use rs232(baud=9600, xmit=PIN_C6, rcv=PIN_C7,ERRORS)
#include <string.h>
#include <input.c>
#include <stdio.h>
#include <stdlib.h>
#include <usb_cdc.h>
#include <nju6355.c>
BYTE get_number() {
char first,second;
do {
first=usb_cdc_getc();
} while ((first<'0') || (first>'9'));
usb_cdc_putc(first);
first-='0';
do {
second=usb_cdc_getc();
} while (((second<'0') || (second>'9')) && (second!='\r'));
usb_cdc_putc(second);
if(second=='\r')
return(first);
else
return((first*10)+(second-'0'));
}
void set_clock(){
BYTE day, mth, year, dow, hour, min;
printf(usb_cdc_putc,"\r\nPress ENTER after 1 digit answers.");
printf(usb_cdc_putc,"\r\nYear 20: ");
year=get_number();
printf(usb_cdc_putc,"\r\nMonth: ");
mth=get_number();
printf(usb_cdc_putc,"\r\nDay: ");
day=get_number();
printf(usb_cdc_putc,"\r\nWeekday 1-7: ");
dow=get_number();
printf(usb_cdc_putc,"\r\nHour: ");
hour=get_number();
printf(usb_cdc_putc,"\r\nMin: ");
min=get_number();
rtc_set_datetime(day,mth,year,dow,hour,min);
printf(usb_cdc_putc,"\r\n\n");
}
void main()
{
char c;
int8 i ;
unsigned char d;
unsigned char key;
int16 results[128];
char cmd;
BYTE day, mth, year, dow, hour, min, sec;
usb_init_cs();
while (TRUE)
{
usb_task();
if (usb_cdc_kbhit())
{
c=usb_cdc_getc();
if (c=='\n') {putc('\r'); putc('\n');}
if (c=='\r') {putc('\r'); putc('\n');}
while(true)
{
ART:
d = usb_cdc_getc();
if(d=='C') // push C for CLOCK
{
while (key!=32) // push SPACE BAR to stop
{
if(usb_cdc_kbhit())
{key=usb_cdc_getc();}
rtc_init();
printf(usb_cdc_putc,"\r\nPress S to change, D to display: ");
do {
cmd=usb_cdc_getc()&0xdf;
} while ((cmd!='D')&&(cmd!='S'));
if(cmd=='S')
set_clock();
printf(usb_cdc_putc,"\r\n");
while (TRUE) {
rtc_get_date( day, mth, year, dow);
rtc_get_time( hour, min, sec );
printf(usb_cdc_putc,"%02u/%02u/%02u %02u:%02u:%02u\r",mth,day,year,hour,min,sec);
delay_ms(250);
}
} key=0; // to initialize 'key' not as SPACE BAR
}
} // For USB format
} // For USB format
} // For USB format
}
|
|
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Fri Feb 10, 2017 11:51 pm |
|
|
Break it up into two programs.
1. Test the nju6355 chip and see if it works. Don't use USB. Just use
an ordinary serial port to display the date and time.
if that works OK, then...
2. Test the USB with constant values inserted by you into the
rtc variables, instead of getting them from the nju6355. Example:
Code: |
// Comment out these two lines.
// rtc_get_date( day, mth, year, dow);
// rtc_get_time( hour, min, sec );
// Substitute your own values:
day = 10;
mth = 2;
year = 17;
hour = 10;
min = 15;
sec = 2;
printf(usb_cdc_putc,"%02u/%02u/%02u %02u:%02u:%02u\r",mth,day,year,hour,min,sec); |
Does it display the correct values ? If not, the USB section is causing
the problem. |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19499
|
|
Posted: Sat Feb 11, 2017 3:36 am |
|
|
You should have the fuse NOPBADEN.
On some compiler versions this won't matter (the compiler automatically turns the ADC 'off' on this port), but on quite a few it does. Unless this is specified, or the ADC is turned off, PortB 'wakes up' setup for analog I/O. Since the 6355 driver uses PortB, this might well be causing it not to work....
Your USB is almost certainly OK, since the '/' and ':' characters are all displaying exactly where they should, and presumably the input code works (it wouldn't if the carriage return wasn't being recognised). I suspect you are not actually talking to the RTC correctly.
If it behaves the same using the standard serial port (as PCM__programmer suggests), then you 'know' that this is where the problem lies.
At heart this makes the fundamental point that always applies when debugging. Trying to debug two things at once, is very unlikely to work. You need to 'know' you have the RTC working _before_ you try to get it going with USB.... |
|
 |
art
Joined: 21 May 2015 Posts: 181
|
|
Posted: Sat Feb 11, 2017 6:04 am |
|
|
Dear ttelmah and pcm
Thanks for your suggestion... I will try standard serial port to test the EX_RTC as suggested |
|
 |
temtronic
Joined: 01 Jul 2010 Posts: 9221 Location: Greensville,Ontario
|
|
Posted: Sat Feb 11, 2017 7:51 am |
|
|
Whenever I see 'odd' numbers like 165,165,165 I immediately think of no pullup resistors on I/O lines or incorrect power.
Have to ask ,are you running the PIC and RTC at 5 volts?
Do you require pullup resistors for the 6355?
As others have said, split the program into 2 and get the RTC 'up and running' first, then deal with the USB communications.
Jay |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19499
|
|
Posted: Sun Feb 12, 2017 11:30 am |
|
|
The 6355 doesn't want pull-ups.
It uses what is effectively 'half duplex' SPI. In fact it could be driven easier and faster using the SPI hardware, and just adding an external transceiver to connect it's single I/O pin to either SDO or SDI based on the status of an extra direction control line. Or it could be driven very easily using the existing #USE SPI setup, and configuring two streams, one 'output only', and one 'input only'. Then set the direction line, and only enable the SPI you want to use. 165, is actually quite an 'odd' but pattern 0b10100101, which either suggests a timing problem, or that there is something wrong with the driver being used. |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|