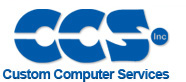 |
 |
View previous topic :: View next topic |
Author |
Message |
ElectronPIC
Joined: 26 Dec 2016 Posts: 9
|
|
Posted: Thu Dec 29, 2016 4:36 pm |
|
|
temtronic wrote: | re: WDT
WDT should be disabled until you're 100% confident your code is correct and 'market ready' THEN enable the WDT and see what happens....
WDT testing could easily take another week or 2....
It needs to be disabled(off) during development as any 'quirk' in your code or even a compiler 'bug' _might_ go 'funny' causing the WDT to trigger when really it's YOUR code , not the WDT that reset the PIC.
Nothing can cause more grief than trying to figure out what's gone 'wrong' when something else leads you to the wrong path or conclusion...
Jay |
Oh, I see! I shouldn't "mask" the origin of the error. Thanks! |
|
 |
ElectronPIC
Joined: 26 Dec 2016 Posts: 9
|
|
Posted: Thu Dec 29, 2016 4:43 pm |
|
|
kieranjefferies wrote: | Hi ElectronPIC, the reason you don't want to disable the RDA Interrupt is basically, you have no control over when bytes of data will arrive at the receiver input and if you don't read in and process / parse these byes before the arrival of subsequent bytes, they are over-written and lost. You could implement a solution without the RDA Interrupt if you had a very low baud rate, delays between subsequent bytes etc and dedicate most of your program resources to monitoring the serial port flags. But once you master the use of the RDA IRQ, you can create elegant and efficient routines which handle all communications reception with minimal impact on your main program.... the reception of data happens in the background. You can bring this to the ultimate level where the main program loop is notified of the reception of a complete & verified packet of data... |
I understand what you're saying. But if I disable the interrupt because / when the receive buffer is full, the characters will be lost regardless of whether I do that or overwrite the last buffer position. It is not like this?. |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19499
|
|
Posted: Fri Dec 30, 2016 2:46 am |
|
|
It doesn't have to be.
Two different approaches:
First the 'circular buffer'. Here the output pointer 'chases' the input one 'round' an imaginary circle of stored data. When a character is received you put it into the 'input' location increment this, and exit.
You can test if there is any data waiting (input != output).
So long as the whole 'processing' takes less time than the incoming data takes to arrive, you can keep going for ever, and not lose anything.
The second is 'toggled buffers'
- simple version just looking for 'linefeed':
Code: |
#defing MAX_LINE 32 //line length to allow
int8 line_buffer[2][MAX_LINE]; //make sizes to suit
int1 input_line=0; //which buffer interrupt is to use
int1 line_seen=FALSE; //flag for a line having been seen
#define OTHER_LINE (input_line^1)
#INT_RDA
void uart1_rx(void)
{
static int8 char_address=0; //start at the beginning of a line
int8 rx_char;
rx_char=fgetc(PORT1); //change to suit your port naming
if (rx_char=='\n')
{
//line feed seen
line_buffer[input_line][char_address]='\0'; //terminate line
input_line = OTHER_LINE; //toggle to other line
line_seen=TRUE; //and flag that line is seen
char_address=0; //start at beginning again
}
else
{
line_buffer[input_line][char_address]=rx_char; //store character
if (++char_address >= (MAX_LINE-1))
--char_address; //keep line from overflowing
}
}
//Then in your main code
if (line_seen) //A complete line has been received
{
line_seen=FALSE;
//Do your parsing here, working on line_buffer[OTHER_LINE][0...31]
}
|
The point about a 'toggle buffer' is you have two 'line' stores, and write to one, until whatever your code wants to use as an 'end of line marker' is seen. Then you set a flag to say 'OK I have a line', and start writing to the other buffer. Your main code then accesses the 'other line' that is not currently being written. This is great for 'line based' input data. As shown, since the 'end of line' marker generates the NULL terminator, standard string functions can be used in the main.
Remember that the buffer will need to be at least one character 'longer' than the line length you are working with (to allow for the terminator). Also as written, it stops incrementing the input pointer if the line tries to get 'too long'.
Now, provided the parser can do it's work quicker than a complete line can ever arrive, nothing need ever be missed. |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|