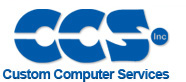 |
 |
View previous topic :: View next topic |
Author |
Message |
benoitstjean
Joined: 30 Oct 2007 Posts: 566 Location: Ottawa, Ontario, Canada
|
Use STREAM 'type' as function parameter |
Posted: Wed Sep 07, 2016 12:22 pm |
|
|
Compiler: 5.026
Device: PIC24HJ256GP206
Quick question...
I am using two UARTS on my PIC: UART1 talks to a serial modem. UART2 displays stuff to a puTTY serial console. The UART configuration is like this:
#use rs232( UART1, baud=115200, parity=N, bits=8, STREAM=MODEM_SERIAL, ERRORS )
#use rs232( UART2, baud=115200, parity=N, bits=8, STREAM=CONSOLE_SERIAL, ERRORS )
UART2 (puTTY console) is used for when I am locally connected to my unit using an FTDI USB-to-SERIAL cable.
UART1 (modem) sends and receives data through the modem's TCP channel when I am connected to the modem via a remote PC.
I am trying to do this:
Code: |
if( IPClient.IsConnected == TRUE )
{
unsigned int8 const Stream = MONITOR_SERIAL;
}
else
{
unsigned int8 const Stream = MODEM_SERIAL;
}
fprintf( Stream, "SYSTEM MENU:" );
|
and I am getting this error at the fprintf line: <unidentified identifier Stream>
So.... How can I pass a 'stream' to the fprintf function? |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Wed Sep 07, 2016 1:27 pm |
|
|
Stream is not a system variable. You can't do it. |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19496
|
|
Posted: Wed Sep 07, 2016 2:32 pm |
|
|
It's worth understanding in some ways what a stream 'is'. It's effectively a pre-processor command to say 'substitute the code for this I/O device into the command'. As such it can't be a variable, since it is changing the code that is actually compiled.
However you can cheat perfectly effectively for this:
Code: |
#use rs232( UART1, baud=115200, parity=N, bits=8, STREAM=MODEM_SERIAL, ERRORS )
#use rs232( UART2, baud=115200, parity=N, bits=8, STREAM=CONSOLE_SERIAL, ERRORS )
#define MODEM 0
#define CONSOLE 1
int8 Target=MODEM;
void routed_putc(char chr)
{
if (Target==MODEM)
fputc(chr,MODEM_SERIAL);
else
fputc(chr,CONSOLE_SERIAL);
}
//Then
if (IPClient.IsConnected==TRUE)
Target=CONSOLE;
else
Target=MODEM;
//When you want to print
printf(routed_putc, "SYSTEM MENU:" );
|
This way two actual putc routines are generated. One for each of the two targets, selected by the value stored in 'Target'. A single printf, is generated, that sends each character to the subroutine that decides which one to routes to. Obviously big saving in not requiring two sets of printf code.
In fact the 'routed_putc', could internally test the IPClient.IsConnected, so the routing becomes completely automatic, and without the need for another variable  |
|
 |
benoitstjean
Joined: 30 Oct 2007 Posts: 566 Location: Ottawa, Ontario, Canada
|
|
Posted: Fri Sep 09, 2016 6:16 am |
|
|
That's what I thought also. Not possible. But using a flag based on if the TCP client is connected or not was also the other solution.
Thanks for all your help! It's appreciated, as always!
Ben |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|