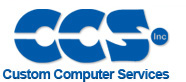 |
 |
View previous topic :: View next topic |
Author |
Message |
jartur32
Joined: 24 Feb 2014 Posts: 10
|
I2C 18f2550 master received string - 16f1503 slave (SOLVED) |
Posted: Fri Mar 21, 2014 1:36 pm |
|
|
Hi all
I'm having a problems when using I2C in one project.
In my project, I receive a data string from i2c master to slave perfect, but I can not send a request to return data from the master to the slave, which only need to return a string to the master pic. I've only gotten back a single character with no problem.
I used PIC18F2550 master and 16f1503 slave.
Thank so much !
Master send code 18f2550
Code: |
#define SDA_PIN PIN_B0
#define SCL_PIN PIN_B1
#use I2C(MASTER, SDA=SDA_PIN, SCL=SCL_PIN, FORCE_HW, FAST)
#define lenbuff_buffer_i2c_incoming 10
char buffer_i2c_incoming[lenbuff_buffer_i2c_incoming];
.
.
.
void main()
{
i2c_start(); // ATTENTION Slaves!
i2c_write(Slave_1_ADDR + 1); // adding 1 to the address tells the slave that you will be reading data from it
buffer_i2c_incoming[0] = i2c_read(1); // read data from the slave (a '1' is default and sends and ACK(acknowledge) to the slave upon successful
buffer_i2c_incoming[1] = i2c_read(1); // read data from the slave (a '1' is default and sends and ACK(acknowledge) to the slave upon successful
buffer_i2c_incoming[2] = i2c_read(1); // read data from the slave (a '1' is default and sends and ACK(acknowledge) to the slave upon successful
buffer_i2c_incoming[3] = i2c_read(1); // read data from the slave (a '1' is default and sends and ACK(acknowledge) to the slave upon successful
buffer_i2c_incoming[4] = i2c_read(1); // read data from the slave (a '1' is default and sends and ACK(acknowledge) to the slave upon successful
buffer_i2c_incoming[6] = i2c_read(0); // a '0' tells the slave that reading time is over and the Master doesn't want any more data
i2c_stop(); // release the bus
delay_ms(1000);
}
|
Slave received code 16f1503
Code: |
#define SCL_i2c PIN_C0
#define SDA_i2c PIN_C1
#USE I2C(SLAVE, sda=SDA_i2c, scl=SCL_i2c, ADDRESS=0x10, FORCE_HW, FAST)
#define lenbuff_string_i2c_return 7
char string_i2c_return[lenbuff_string_i2c_return] = {'1','2','3','4','5','6','\0'};
.
.
.
#INT_SSP
void ssp_interrupt()
{
char state,incoming;
state = i2c_isr_state();
if(state < 0x80)//Master send data
{
incoming = i2c_read();//An array will be needed to store data if more than one byte is transferred
if (state == 0)
count_i2c_data = 0; //here need to read but not store
else
{
string_i2c[count_i2c_data++] = incoming;
if (incoming==0)
FLAG_have_string=TRUE;
}
}
else
if(state == 0x80)//Master need data
{
for (int8 j = 0; j <= strlen(string_i2c_return); j++) // for
i2c_write(string_i2c_return[j]); // send actual data
}
}
|
Last edited by jartur32 on Sat Mar 22, 2014 6:59 pm; edited 2 times in total |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Fri Mar 21, 2014 2:44 pm |
|
|
Are you aware that you have a previous, from Feb. 23, 2014, where you
ask the exact same question and Ttelmah solved it for you with sample
code, and you tested it and declared the problem solved ?
http://www.ccsinfo.com/forum/viewtopic.php?t=51961 |
|
 |
jartur32
Joined: 24 Feb 2014 Posts: 10
|
|
Posted: Fri Mar 21, 2014 3:11 pm |
|
|
hi pcm programmer
This is a new problem, I can not make the pic slave responds to the pic master with a string, and the pic-master receives the string data to show value on LCD. I've only gotten back a single character to the master. il change the topic name to one more correct and diferent
Last edited by jartur32 on Fri Mar 21, 2014 3:25 pm; edited 1 time in total |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Fri Mar 21, 2014 3:24 pm |
|
|
Right, but in your new code here you are attempting to send the whole
string in one pass, inside the isr. Try it with the slave sending one byte
per #int_ssp interrupt.
Also, you are declaring locals inside the code. This isn't really supported
in CCS. I mean this:
You should declare locals at the start of the function. |
|
 |
jartur32
Joined: 24 Feb 2014 Posts: 10
|
|
Posted: Sat Mar 22, 2014 6:58 pm |
|
|
Thank pcm programmer
Final code
Master
Code: |
#define SDA_PIN PIN_B0
#define SCL_PIN PIN_B1
#use I2C(MASTER, SDA=SDA_PIN, SCL=SCL_PIN, FORCE_HW, FAST)
#define ADDR 0x10
#define lenbuff_i2c 6
char buffer_i2c[lenbuff_i2];
void read_i2c(void)
{
i2c_start(); // ATTENTION Slaves!
i2c_write(ADDR); // address slave
i2c_write(0x00); // address data 0
i2c_start(); // ATTENTION Slaves!
i2c_write(ADDR + 1); // Adding 1 to the address reading data
buffer_i2c[0] = i2c_read(1); // Read data from the slave
buffer_i2c[1] = i2c_read(1); // Read data from the slave
buffer_i2c[2] = i2c_read(1); // Read data from the slave
buffer_i2c[3] = i2c_read(1); // Read data from the slave
buffer_i2c[4] = i2c_read(0); // A '0' Master doesn't want any more data
buffer_i2c[5] = '\0';
i2c_stop();
}
|
Slave
Code: |
#define SCL_i2c PIN_C0
#define SDA_i2c PIN_C1
#USE I2C(SLAVE, sda=SDA_i2c, scl=SCL_i2c, ADDRESS=0x10, FORCE_HW, FAST)
int8 j=0;
int1 FLAG_have_string = FALSE;
#define lenbuff_string_i2c 7
char string_i2c[lenbuff_string_i2c];
#define lenbuff_string_i2c_return 6
char string_i2c_return[lenbuff_string_i2c_return];
#INT_SSP
void ssp_interrupt()
{
char state,incoming;
state = i2c_isr_state();
if(state < 0x80)//Master envia datos
{
incoming = i2c_read();//An array will be needed to store data if more than one byte is transferred
if (state == 0)
{
count_i2c_data = 0; //here need to read but not store
j = 0;
}
else
{
string_i2c[count_i2c_data++] = incoming;
if ( incoming == 0 )
FLAG_have_string=TRUE;
}
}
if(state >= 0x80)//Master pide datos
{
i2c_write(string_i2c_return[j++]); // send actual data
}
}
|
|
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|