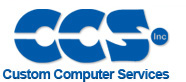 |
 |
View previous topic :: View next topic |
Author |
Message |
Mike Walne
Joined: 19 Feb 2004 Posts: 1785 Location: Boston Spa UK
|
|
Posted: Fri Jun 14, 2013 10:53 am |
|
|
OK. So you're using the outputs 0-4 of the 4017 to drive the matrix anodes.
You'll run into problems with this approach.
You've got issues with code layout and the code itself.
First the code layout:-
I've re-arranged part of your code to see where all the loops are.
Code: |
// ADD ALL PIC TRIS SETUP
setup_comparator(NC_NC_NC_NC); // Comparator OFF
set_tris_a(0);
set_tris_b(0);
delay_ms(500);
while (TRUE)
{
for (i = 0; i <5; i++)
{
port_b = i;
port_b=mask[i];
delay_ms(25);
{
// Clock 4017
output_high(PIN_A0);
delay_ms(5);
output_low(PIN_A0);
delay_ms(5);
}
}
// Reset 4017
output_high(PIN_A1);
delay_ms(5);
output_low(PIN_A1);
delay_ms(5);
} //End while
}
|
You don't need any of these lines. Code: |
// ADD ALL PIC TRIS SETUP
setup_comparator(NC_NC_NC_NC); // Comparator OFF
set_tris_a(0);
set_tris_b(0);
| You're not using the CCP module.
The CCS compiles takes care of the tris states most of the time.
It's very rare for you to have to get involved.
What is this line for?
Use the CCS output function rather than this line
You don't need the braces round this section of code. Code: | {
// Clock 4017
output_high(PIN_A0);
delay_ms(5);
output_low(PIN_A0);
delay_ms(5);
} |
Then the code itself:-
You're delays are far too long in the loop.
You've got a total delay_ms(x) of some 185ms in your loop.
The display must be flickering something rotten.
To get a reasonable display your loop needs to take no more than 20ms.
(10ms would be better)
And you're doing things in the wrong sequence.
To avoid ghosting, the display must be ALL OFF when you clock (or reset) the 4017.
Mike |
|
 |
wala.iswara
Joined: 10 Jun 2013 Posts: 21
|
|
Posted: Fri Jun 14, 2013 10:24 pm |
|
|
Hallo Mike,
Here is my schematic.
Code: |
_____
-[ ]->Q1----------------------------------------------------------
-[ ]->Q2------------------------------------------------------- :
-[ 4 ]->Q3---------------------------------------------------- : :
-[ 0 ]->Q4------------------------------------------------- : : :
-[ 1 ]->Q5---------------------------------------------- : : : :
RA0>--Clk--[ 7 ]- : : : : :
RA1>--Rst--[ ]- : : : : :
-[_____]- : : : : :
: : : : :
7 330 R : _ : : :
RB6<-----------------/WWW\-------------------------------O I I O O
RB5<-----------------/WWW\-------------------------------I I I O O
_
RB4<-----------------/WWW\-------------------------------O I I O O
_
RB3<-----------------/WWW\-------------------------------O I I O O
_
RB2<-----------------/WWW\-------------------------------O I I O O
_
RB1<-----------------/WWW\-------------------------------O I I O O
RB0<-----------------/WWW\-------------------------------I I I I I
_
I is unexpected result.. |
// output PORTB is i?
Like what? Example please.
Code: | {
// Clock 4017
output_high(PIN_A0);
delay_ms(5);
output_low(PIN_A0);
delay_ms(5);
} | Ok, i will try.
Yes, my delay is too long. And the LED is blinking. And i have unexpected result as my circuit above. |
|
 |
Mike Walne
Joined: 19 Feb 2004 Posts: 1785 Location: Boston Spa UK
|
|
Posted: Sat Jun 15, 2013 2:43 am |
|
|
You don't need this line it serves no purpose
What you actually need is the next line
Which should be replaced with something like (untested)
Then you don't need this line at the head of your code
It's not portable between processors.
Quote: | And i have unexpected result as my circuit above. |
You do not have unexpected behaviour.
I told you what was wrong in my previous post. Quote: |
And you're doing things in the wrong sequence.
To avoid ghosting, the display must be ALL OFF when you clock (or reset) the 4017. |
To understand what's happening play at being computer.
Write down the states of all the relevant registers, ports etc.
Step through the code to the next delay_ms(x), write them all down again.
Take note of the time spent in each state.
Do this 'til you've been through the whole sequence (or until the penny drops).
Mike
PS You SHOULD find the 4017 spends significant time in SIX states not the FIVE that you intend!
EDIT
You've got ghosting on a grand scale!
There is loads on this forum covering MUX displays.
Makes no difference whether it's dot matrix or seven seg., the basics are the same.
Do some searching and reading.
Try to work it out for yourself, then you won't keep makng the same errors. |
|
 |
wala.iswara
Joined: 10 Jun 2013 Posts: 21
|
|
Posted: Mon Jun 17, 2013 7:48 am |
|
|
Hallo,
I see all of my LED matrix emitting with code below. But i want to display 1.
Code: | #include <16F628.h>
#fuses XT,NOWDT,NOPUT,NOPROTECT,NOBROWNOUT,NOLVP
#use delay(clock=4000000)
#define Clock_4017 PIN_A0 // -> to pin_14 4017
#define Reset_4017 PIN_A1 // -> to pin_15 4017
int8 number[5]= { 0b00000001, 0b00000001, 0b01111111, 0b00100001, 0b00100001 }; // 1
void main(){
int8 i;
// ADD ALL PIC TRIS SETUP
setup_comparator(NC_NC_NC_NC); // Comparator OFF
set_tris_a(0);
set_tris_b(0);
delay_ms(500);
while (TRUE){
for (i = 0; i <5; i++) {
output_b(0b00000000);
output_b(number[i]);
// Clock 4017
output_low(PIN_A0);
output_high(PIN_A0);
delay_us(6);
output_b(0b00000000);
} //End while
} |
Is it caused by the delay or the code?  |
|
 |
Mike Walne
Joined: 19 Feb 2004 Posts: 1785 Location: Boston Spa UK
|
|
Posted: Mon Jun 17, 2013 4:57 pm |
|
|
I'm assuming you've not changed the hardware.
Why are you no longer clearing the 4017?
I told you to turn ALL the LEDs OFF whilst either clocking or clearing the 4017; you're now turning ALL the LEDs ON when clocking.
Mike
PS
You need to be certain of the 4017 state.
As it stands you've no idea where it is.
You really need to get back to your original circuit WITHOUT the 4017.
Slightly modified, it SHOULD have been a lot simpler!!!
I.e. with PORT B driving the anodes, port A pulling the cathodes down.
(The A4 open drain O/P would then not be a problem.) |
|
 |
wala.iswara
Joined: 10 Jun 2013 Posts: 21
|
|
Posted: Mon Jun 17, 2013 7:41 pm |
|
|
Dear Mike,
I will keep my circuit with decade counter, and will use shift register in future.
But, will my MCU broken if i write, delete, and rewrite again in many of time?
I read the data sheet like this:
• High Endurance FLASH/EEPROM Cell
- 100,000 write FLASH endurance
- 1,000,000 write EEPROM endurance
- 100 year data retention
This my new code.
Code: | #include <16F628.h>
#fuses XT,NOWDT,NOPUT,NOPROTECT,NOBROWNOUT,NOLVP
#use delay(clock=4000000)
#define Clock_4017 PIN_A0 // -> to pin_14 4017
#define Reset_4017 PIN_A1 // -> to pin_15 4017
int8 number[5]= { 0b00000001, 0b00000001, 0b01111111, 0b00100001, 0b00100001 }; // 1
void main(){
int8 i;
// ADD ALL PIC TRIS SETUP
setup_comparator(NC_NC_NC_NC); // Comparator OFF
set_tris_a(0);
set_tris_b(0);
delay_ms(500);
while (TRUE){
for (i = 0; i <5; i++) {
output_b(number[i]);
// Clock 4017
output_high(PIN_A0);
output_low(PIN_A0);
delay_ms(6);
// Reset 4017
output_high(PIN_A1);
output_low(PIN_A1);
output_b(0b00000000);
} //End while
} | [/code] |
|
 |
oxo
Joined: 13 Nov 2012 Posts: 219 Location: France
|
|
Posted: Tue Jun 18, 2013 2:25 am |
|
|
I think you should give up Mike. |
|
 |
Mike Walne
Joined: 19 Feb 2004 Posts: 1785 Location: Boston Spa UK
|
|
Posted: Tue Jun 18, 2013 2:40 am |
|
|
You're not making much progress are you?
Try the code below.
I've done it for a 4017 using Q0-Q4.
Use the optional clock pulse for your Q1-Q5 connection.
I don't have your processor.
Change the include to your PIC.
Code: |
#include <16F876a.h>
#fuses XT,NOWDT,NOPUT,NOPROTECT,NOBROWNOUT,NOLVP
#use delay(clock=4000000)
#define Clock_4017 PIN_A0 // -> to pin_14 4017
#define Reset_4017 PIN_A1 // -> to pin_15 4017
#define Max_Col 5
int8 mask[]= { 0xFE, 0xFE, 0x00, 0xDE, 0xDE }; // 1
int8 col;
void main()
{
delay_ms(500);
while (TRUE)
{
// Reset 4017. system then in known state
output_b(0xff); // force all LEDs OFF
output_high(Reset_4017);
output_low(Reset_4017);
// Optional Clock 4017
//output_high(Clock_4017);
//output_low(Clock_4017);
for (col = 0; col <Max_Col; col++)
{
output_b(mask[col]);
delay_ms(2);
// Clock 4017
output_b(0xff); // force all LEDs OFF
output_high(Clock_4017);
output_low(Clock_4017);
}
} //End while
} |
Tell me how you get on with this code
Mike |
|
 |
wala.iswara
Joined: 10 Jun 2013 Posts: 21
|
|
Posted: Tue Jun 18, 2013 6:48 am |
|
|
Dear Mike.
Sorry was make you busy.
My code work fine, clear and bright. Just blinking coused by the delay.
But i will try your code.
here my new code.
Code: | #include <16F628.h>
#fuses XT,NOWDT,NOPUT,NOPROTECT,NOBROWNOUT,NOLVP
#use delay(clock=4000000)
#define Clock_4017 PIN_A0 // -> to pin_14 4017
#define Reset_4017 PIN_A1 // -> to pin_15 4017
int8 number[5]= { 0b11111110, 0b11111110, 0b10000000, 0b11011110, 0b11011110 }; // 1
void main(){
int8 i;
// ADD ALL PIC TRIS SETUP
setup_comparator(NC_NC_NC_NC); // Comparator OFF
set_tris_a(0);
set_tris_b(0);
delay_ms(500);
while (TRUE){
// Reset 4017 and clearing.
output_high(PIN_A1);
output_low(PIN_A1);
// Displaying number[5]
for (i = 0; i <5; i++) {
output_b(number[i]);
// Clock 4017
output_high(PIN_A0);
delay_ms(6);
output_low(PIN_A0);
delay_ms(6);
output_a(0b00000000);
output_b(0b00000000);
}
} //End while
} |
|
|
 |
Mike Walne
Joined: 19 Feb 2004 Posts: 1785 Location: Boston Spa UK
|
|
Posted: Tue Jun 18, 2013 2:23 pm |
|
|
This topic is going nowhere.
Others and I have shown you how to systematically achieve your goal.
You're simply wasting everybody's time by making seemingly uninformed stabs in the dark, and ignoring advice you've been given.
I've had enough, I'm out.
Mike |
|
 |
Gabriel
Joined: 03 Aug 2009 Posts: 1067 Location: Panama
|
for what its worth... |
Posted: Tue Jun 18, 2013 4:23 pm |
|
|
circa 2006....school project...it worked quite good..
Please note this was my first CCS code...
it drives a 5*7 matrix...
Code: | //*****************************************************************************
//
// Gabriel Barrios
// date: 12/11/2006
//
// controls a LED dot matrix to display standard ASCII characters
// the pic receives a character serially and forwards the current
// character once its received a new one. in order to display the
// the proper pattern it searches for the characters pattern on its
// data table using a binary search based algorithm
//
//*****************************************************************************
#if defined(__PCB__)
#include <16f877a.h>
#fuses HS,NOWDT,NOPROTECT
#use delay(clock=20000000) // must be 20 MHz
#elif defined(__PCM__)
#include <16f877a.h>
#fuses HS,NOWDT,NOPROTECT,NOLVP
#use delay(clock=20000000) // must be 20 MHz
#use fast_io(A)
#use fast_io(B)
#use rs 232(baud=9600, xmit=PIN_C6, rcv=PIN_C7) // serial
void Find();
int Area_numbers();
int Area_LoCase_top1();
int Area_LoCase_top2();
int Area_LoCase_low1();
int Area_LoCase_low2();
int Area_HiCase_top1();
int Area_HiCase_top2();
int Area_HiCase_low1();
int Area_HiCase_low2();
char key,next;
char *uart_flag;
int presente;
int col1,col2,col3,col4,col5;
void main()
{
SET_TRIS_A(0x00);
SET_TRIS_B(0x00);
enable_interrupts(INT_RDA);
enable_interrupts(GLOBAL);
*uart_flag=0x18;
presente=0;
key =' ';
col1 = 0;
col2 = 0;
col3 = 0;
col4 = 0;
col5 = 0;
while(presente==0)
{
}
if(presente==1)
presente=0;
find();
}
void find()
{
while(1)
{
if((key>='a')&&(key<='z')) //lower case preselection
{
if((key>='a')&&(key<='g'))
Area_LoCase_top1();
if((key>='h')&&(key<='n'))
Area_LoCase_top2();
if((key>='o')&&(key<='t'))
Area_LoCase_low1();
if((key>='u')&&(key<='z'))
Area_LoCase_low2();
}
if((key>='A')&&(key<='Z')) //higer case preselection
{
if((key>='A')&&(key<='G'))
Area_HiCase_top1();
if((key>='H')&&(key<='N'))
Area_HiCase_top2();
if((key>='O')&&(key<='T'))
Area_HiCase_low1();
if((key>='U')&&(key<='Z'))
Area_HiCase_low2();
}
if((key>='0')&&(key<='9'))
Area_numbers();
next = key; // prestore value to send to next module
Loop: // display
output_a(0x01);
output_b(col1);
delay_ms(1);
output_b(0x00);
output_a(0x02);
output_b(col2);
delay_ms(1);
output_b(0x00);
output_a(0x04);
output_b(col3);
delay_ms(1);
output_b(0x00);
output_a(0x08);
output_b(col4);
delay_ms(1);
output_b(0x00);
output_a(0x10);
output_b(col5);
delay_ms(1);
output_b(0x00);
if(presente==0) goto Loop;
presente=0;
putchar(next);
}
}
int Area_numbers()
{
if(key=='1')goto L_1;
if(key=='2')goto L_2;
if(key=='3')goto L_3;
if(key=='4')goto L_4;
if(key=='5')goto L_5;
if(key=='6')goto L_6;
if(key=='7')goto L_7;
if(key=='8')goto L_8;
if(key=='9')goto L_9;
if(key=='0')goto L_0;
L_1: // number 1
col1=0x00;
col2=0x40;
col3=0xFF;
col4=0x42;
col5=0x00;
return(0);
L_2: // number 2
col1=0x46;
col2=0x49;
col3=0x51;
col4=0x61;
col5=0x42;
return(0);
L_3: // number 3
col1=0x31;
col2=0x4B;
col3=0x45;
col4=0x41;
col5=0x21;
return(0);
L_4: // number 4
col1=0x10;
col2=0xFF;
col3=0x12;
col4=0x14;
col5=0x18;
return(0);
L_5: // number 5
col1=0x39;
col2=0x45;
col3=0x45;
col4=0x45;
col5=0x27;
return(0);
L_6: // number 6
col1=0x30;
col2=0x49;
col3=0x49;
col4=0x4A;
col5=0x3C;
return(0);
L_7: // number 7
col1=0x03;
col2=0x05;
col3=0x09;
col4=0x71;
col5=0x01;
return(0);
L_8: // number 8
col1=0x36;
col2=0x49;
col3=0x49;
col4=0x49;
col5=0x36;
return(0);
L_9: // number 9
col1=0x1E;
col2=0x29;
col3=0x49;
col4=0x49;
col5=0x06;
return(0);
L_0: // number 0
col1=0x3E;
col2=0x45;
col3=0x49;
col4=0x51;
col5=0x3E;
return(0);
}
int Area_LoCase_top1()
{
if(key=='a')goto L_a;
if(key=='b')goto L_b;
if(key=='c')goto L_c;
if(key=='d')goto L_d;
if(key=='e')goto L_e;
if(key=='f')goto L_f;
if(key=='g')goto L_g;
L_a: // low case a
col1=0x78;
col2=0x54;
col3=0x54;
col4=0x54;
col5=0x20;
return(0);
L_b: // low case b
col1=0x30;
col2=0x48;
col3=0x48;
col4=0x48;
col5=0xFF;
return(0);
L_c: // low case c
col1=0x44;
col2=0x44;
col3=0x44;
col4=0x44;
col5=0x38;
return(0);
L_d: // low case d
col1=0xFF;
col2=0x48;
col3=0x48;
col4=0x48;
col5=0x30;
return(0);
L_e: // lwo case e
col1=0x08;
col2=0x54;
col3=0x54;
col4=0x54;
col5=0x38;
return(0);
L_f: // low case f
col1=0x09;
col2=0x09;
col3=0xFE;
col4=0x08;
col5=0x08;
return(0);
L_g: // low case g
col1=0x3C;
col2=0x54;
col3=0x54;
col4=0x54;
col5=0x08;
return(0);
}
int Area_LoCase_top2()
{
if(key=='h')goto L_h;
if(key=='i')goto L_i;
if(key=='j')goto L_j;
if(key=='k')goto L_k;
if(key=='l')goto L_l;
if(key=='m')goto L_m;
if(key=='n')goto L_n;
L_h: // low case h
col1=0x70;
col2=0x08;
col3=0x08;
col4=0x10;
col5=0xFF;
return(0);
L_i: // low case i
col1=0x00;
col2=0x40;
col3=0xFD;
col4=0x44;
col5=0x00;
return(0);
L_j: // low case j
col1=0x00;
col2=0x3D;
col3=0x44;
col4=0x40;
col5=0x20;
return(0);
L_k: // low case k
col1=0x44;
col2=0x28;
col3=0x10;
col4=0xFF;
col5=0x00;
return(0);
L_l: // lwo case l
col1=0x00;
col2=0x40;
col3=0xFF;
col4=0x41;
col5=0x00;
return(0);
L_m: // low case m
col1=0xF8;
col2=0x04;
col3=0xF8;
col4=0x04;
col5=0xFC;
return(0);
L_n: // low case n
col1=0xF8;
col2=0x04;
col3=0x04;
col4=0x08;
col5=0xFC;
return(0);
}
int Area_LoCase_low1()
{
if(key=='o')goto L_o;
if(key=='p')goto L_p;
if(key=='q')goto L_q;
if(key=='r')goto L_r;
if(key=='s')goto L_s;
if(key=='t')goto L_t;
L_o: // low case o
col1=0x38;
col2=0x44;
col3=0x44;
col4=0x44;
col5=0x38;
return(0);
L_p: // low case p
col1=0x08;
col2=0x14;
col3=0x14;
col4=0x14;
col5=0xFC;
return(0);
L_q: // low case q
col1=0xFC;
col2=0x14;
col3=0x14;
col4=0x14;
col5=0x08;
return(0);
L_r: // low case r
col1=0x04;
col2=0x04;
col3=0x08;
col4=0xFC;
col5=0x00;
return(0);
L_s: // lwo case s
col1=0x20;
col2=0x54;
col3=0x54;
col4=0x54;
col5=0X48;
return(0);
L_t: // low case t
col1=0x48;
col2=0x48;
col3=0x3F;
col4=0x08;
col5=0x08;
return(0);
}
int Area_LoCase_low2()
{
if(key=='u')goto L_u;
if(key=='v')goto L_v;
if(key=='w')goto L_w;
if(key=='x')goto L_x;
if(key=='y')goto L_y;
if(key=='z')goto L_z;
L_u: // low case u
col1=0x00;
col2=0x00;
col3=0x00;
col4=0x00;
col5=0x00;
return(0);
L_v: // low case v
col1=0x00;
col2=0x00;
col3=0x00;
col4=0x00;
col5=0x00;
return(0);
L_w: // low case w
col1=0x00;
col2=0x00;
col3=0x00;
col4=0x00;
col5=0x00;
return(0);
L_x: // low case x
col1=0x00;
col2=0x00;
col3=0x00;
col4=0x00;
col5=0x00;
return(0);
L_y: // low case y
col1=0x00;
col2=0x00;
col3=0x00;
col4=0x00;
col5=0x00;
return(0);
L_z: // lwo case z
col1=0x00;
col2=0x00;
col3=0x00;
col4=0x00;
col5=0x00;
return(0);
}
//*************************************************************************
int Area_HiCase_top1()
{
if(key=='A')goto L_A;
if(key=='B')goto L_B;
if(key=='C')goto L_C;
if(key=='D')goto L_D;
if(key=='E')goto L_E;
if(key=='F')goto L_F;
if(key=='G')goto L_G;
L_A: // hi case A
col1=0x7E;
col2=0x11;
col3=0x11;
col4=0x11;
col5=0x7E;
return(0);
L_B: // hi case B
col1=0x36;
col2=0x49;
col3=0x49;
col4=0x49;
col5=0xFF;
return(0);
L_C: // hi case C
col1=0x22;
col2=0x41;
col3=0x41;
col4=0x41;
col5=0x3E;
return(0);
L_D: // hi case D
col1=0x1C;
col2=0x22;
col3=0x41;
col4=0x41;
col5=0xFF;
return(0);
L_E: // hi case E
col1=0x41;
col2=0x49;
col3=0x49;
col4=0x49;
col5=0xFF;
return(0);
L_F: // hi case F
col1=0x01;
col2=0x09;
col3=0x09;
col4=0x09;
col5=0xFF;
return(0);
L_G: // hi case G
col1=0x7A;
col2=0x49;
col3=0x49;
col4=0x41;
col5=0x7E;
return(0);
}
int Area_HiCase_top2()
{
if(key=='H')goto L_H;
if(key=='I')goto L_I;
if(key=='J')goto L_J;
if(key=='K')goto L_K;
if(key=='L')goto L_L;
if(key=='M')goto L_M;
if(key=='N')goto L_N;
L_H: // hi case H
col1=0xFF;
col2=0x08;
col3=0x08;
col4=0x08;
col5=0xFF;
return(0);
L_I: // hi case I
col1=0x00;
col2=0x41;
col3=0xFF;
col4=0x41;
col5=0x00;
return(0);
L_J: // hi case J
col1=0x01;
col2=0x3F;
col3=0x41;
col4=0x40;
col5=0x20;
return(0);
L_K: // hi case K
col1=0x41;
col2=0x22;
col3=0x14;
col4=0x08;
col5=0xFF;
return(0);
L_L: // hi case L
col1=0x40;
col2=0x40;
col3=0x40;
col4=0x40;
col5=0xFF;
return(0);
L_M: // hi case M
col1=0xFF;
col2=0x02;
col3=0x0C;
col4=0x02;
col5=0xFF;
return(0);
L_N: // hi case N
col1=0xFF;
col2=0x10;
col3=0x08;
col4=0x04;
col5=0xFF;
return(0);
}
int Area_HiCase_low1()
{
if(key=='O')goto L_O;
if(key=='P')goto L_P;
if(key=='Q')goto L_Q;
if(key=='R')goto L_R;
if(key=='S')goto L_S;
if(key=='T')goto L_T;
L_O: // hi case O
col1=0x3E;
col2=0x41;
col3=0x41;
col4=0x41;
col5=0x3E;
return(0);
L_P: // hi case P
col1=0x06;
col2=0x09;
col3=0x09;
col4=0x09;
col5=0xFF;
return(0);
L_Q: // hi case Q
col1=0x5E;
col2=0x21;
col3=0x51;
col4=0x41;
col5=0x3E;
return(0);
L_R: // hi case R
col1=0x46;
col2=0x29;
col3=0x19;
col4=0x09;
col5=0xFF;
return(0);
L_S: // hi case S
col1=0x31;
col2=0x49;
col3=0x49;
col4=0x49;
col5=0x46;
return(0);
L_T: // hi case T
col1=0x01;
col2=0x01;
col3=0xFF;
col4=0x01;
col5=0x01;
return(0);
}
int Area_HiCase_low2()
{
if(key=='U')goto L_U;
if(key=='V')goto L_V;
if(key=='W')goto L_W;
if(key=='X')goto L_X;
if(key=='Y')goto L_Y;
if(key=='Z')goto L_Z;
L_U: // hi case U
col1=0x3F;
col2=0x40;
col3=0x40;
col4=0x40;
col5=0x3F;
return(0);
L_V: // hi case V
col1=0x1F;
col2=0x20;
col3=0x40;
col4=0x20;
col5=0x1F;
return(0);
L_W: // hi case W
col1=0x3F;
col2=0x40;
col3=0x38;
col4=0x40;
col5=0x3F;
return(0);
L_X: // hi case X
col1=0x63;
col2=0x14;
col3=0x08;
col4=0x14;
col5=0x63;
return(0);
L_Y: // hi case Y
col1=0x07;
col2=0x08;
col3=0x70;
col4=0x08;
col5=0x07;
return(0);
L_Z: // hi case Z
col1=0x43;
col2=0x45;
col3=0x49;
col4=0x51;
col5=0x61;
return(0);
}
#INT_RDA
void serialint()
{
key=getchar();
*uart_flag=(*uart_flag) & (0xEF);
*uart_flag=(*uart_flag) | (0x10);
presente = 1;
} |
_________________ CCS PCM 5.078 & CCS PCH 5.093 |
|
 |
wala.iswara
Joined: 10 Jun 2013 Posts: 21
|
|
Posted: Tue Jun 18, 2013 7:13 pm |
|
|
Hallo,
@oxo:
Hai oxo.
@ Gabriel:
Thanks.
@ Mike:
I just want to start to counting the number Mike. Please don't go any where, just check. Ignoring oxo, but i will try your code to day.
I will try to give the jump statement in my code or Mike's code, as with 7 segment counter counting the number. Mike? |
|
 |
oxo
Joined: 13 Nov 2012 Posts: 219 Location: France
|
|
 |
wala.iswara
Joined: 10 Jun 2013 Posts: 21
|
|
 |
Mike Walne
Joined: 19 Feb 2004 Posts: 1785 Location: Boston Spa UK
|
|
Posted: Wed Jun 19, 2013 9:49 am |
|
|
In previous posts you were advised to read up on multiplex displays.
You either didn't, or could not understand how to adapt to your circuit.
So, I'll explain for your 4017 version.
The main loop needs to go round in <20ms.
Pseudo code for main loop goes roughly like this.
1) Turn ALL cathodes OFF. (i.e. in your case output_b(0xff); )
2) Reset 4017.
3) Turn on correct cathodes for currently active column.
4) Wait for say ~2ms.
5) Turn ALL cathodes OFF. (As before.)
6) Clock 4017.
7) Loop to (3) for all columns.
8) Loop to (1) for ever.
Loop 1-8 is a while(1) loop.
Loop 3-7 is a for(x;y;z) loop.
I've shown you how to set out code in a clearer fashion, you've avoided doing that.
I'm advising you to turn ALL cathodes OFF before changing anode drives.
Again you're studiously avoiding this. I'm saying it because it's good practice and to prevent ghosting issues later.
You titled your work 7x5 matrix.
On all your photos I can only count 4 columns. Which is correct?
Which columns are you actually using, (or think you're using)?
Your photo of the "working" device shows that you're seriously overloading the 4017!!!
Which 4017 version have you got? (4000 B series or High Speed?)
Either way you need to ponder why the full column of lit diodes is significantly less bright than the other lit diodes.
(There's a reason for it. You'll find the answer in the data sheet)
(On a good display, ALL lit diodes should be the brighness)
At this stage you are nowhere near ready to progress to getting your display to show other digits, never mind counting 0 to 9.
Mike |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|