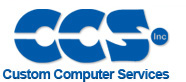 |
 |
View previous topic :: View next topic |
Author |
Message |
ze.vana
Joined: 11 Jun 2011 Posts: 15
|
PIC to PIC Display data transfer |
Posted: Sat May 26, 2012 6:44 am |
|
|
Hi people
that is a simple example of data transfer PIC to PIC using small and cheap PICs. Maybe this would be useful for beginners like me!
send.c
Code: |
#include <16f628a.h>
#fuses INTRC_IO, NOLVP, NOWDT, PUT, BROWNOUT
#use delay (clock=4M)
#use rs232(baud=9600,XMIT=PIN_B2,RCV=PIN_B1,bits=8)
//#include "ds18b20.c"
byte send[3];
#define sreset (pin_b0)//RB0 ->- PIN1 MLCR 16F876 (resets display)
int8 div;
int8 temp=15;//temperature
int8 hrs=7,min=22;
void send_dat(void)
{
int i;
for (i=0; i<3; ++i)//1° RODA O I 10 VEZES
{
putc(send[i]);
}
}
void main(void)
{
int1 powerup;
set_tris_a(0x00); //DQ PIN_a4
set_tris_b(0b00111000); //RB1 RB2 Rx/tx) / RB0 ->- PIN1 MLCR 16F876
output_low sreset;div=0;
powerup=false;
while(true)
{
// ds1307_get_time(hrs,min,sec);
output_toggle(pin_b3);//it's working!
send[0]=hrs; send[1]=min; send[2]=temp;
div++;
if(!powerup && div >=10) { powerup=true; output_high sreset;} //RB0 ->- PIN1 MLCR 16F876
if(powerup && div >10) {send_dat(); div=0; }
}
}
//RESET is to synchronize data transfer during initialization.
///////////////////////////////////////////////////////////////
// Put a 10K resistor from pin1 F876 to VDD 5V //
// to VDD //
// ---------- --------- ------- //
// | RB0 |6-----------1|MCLR B0|- A| DIS- | //
// | F628 | | F876 B1|- B|PLAY | //
// | X RB2|8----------18|C7 RX B2|- C| | //
// | | TXD-> | B3|- D| | //
///////////////////////////////////////////////////////////////
|
Receive.c
Code: |
#include <16F876a.h>
#device ADC=10
#fuses XT, NOLVP, NOWDT, PUT, BROWNOUT
#use delay (clock=4M)
#use rs232(baud=9600,XMIT=PIN_C6,RCV=PIN_C7,bits=8)
int16 div;
int hrs,min;
int hor4,hor3,hor2,hor1;
int temp;
int var2=0, var1=0;
int8 scan,div2;
#include "display.c"
byte receiv[3];
#INT_RDA
void rda_isr()
{
int i;
for (i=0; i<3; ++i)//receive 3 datas
{ //0 is the first
receiv[i]=getc();
}
hrs=receiv[0]; min=receiv[1]; temp=receiv[2];
}
void select(void)
{
if (div <4000 )//show hours
{
dig4=hor4;//dez hora
dig3=hor3;//unid hora
dig2=hor2;//dez minuto
dig1=hor1;//unid minuto
}
if(div >2000 )//show degrees
{
dig4=var2; //dezena de graus
dig3=var1; //unidade de graus
dig2=10; //grau Simbolo
dig1=11;//11= zero off
}
div++;
if(div >4000) div=0;
}
void convert(int valor)//temperature
{
var2=valor/10; // 45 / 10=4
var1=valor -(var2 * 10); // 45 -(4 x 10)=5
}
void main()
{
enable_interrupts(global);
enable_interrupts(INT_rda);
set_tris_a(0x01);
set_tris_b(0x00);
set_tris_C(0b10000000 );
div=0; div2=0; scan=100; //display time -> delay_ms(scan)
while (true)
{
hor4=hrs/10; //ex.: 23 /10 =2
hor3=hrs -(hor4 * 10); // 23 -(2 x 10)=3
hor2=min/10; // ex.: 45 / 10 =4
hor1=min -(hor2 * 10);// 45 -(4 x 10) =5
convert(temp);
mostra();//Display
select();
}
}
|
Display.c
Code: |
////////////////////////////// display moto
//display PIC 16f876a // I use it on my Byke
//B0 A C0 K1 // to show time and degrees
//B1 B C1 K2 //
//B2 C D2 K3 //
//B3 D D3 K4 //
//B4 E //
//B5 F //
//B6 G B7 NOT USED //
//////////////////////////////
struct cont_pins
{
int data:4;//digitos
} cont;
#byte cont = 7 // 7 is the portc address.
//---------------------------------------
byte CONST seg[] = {0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f,0b1100011,0b0000000,0b1000000};
int dig4=0,dig3=0,dig2=0,dig1=0; // 10=degree symbol 11=zero_off 12=negative signal
void mostra(void)
{
output_b(seg[dig4]);
cont.data=0b1110;//1 k4,k3,k2,k1
delay_us(scan);
cont.data=0b1111; //Apaga display.
delay_us(10);
output_b(seg[dig3]);
cont.data=0b1101; //2
delay_us(scan);
cont.data=0b1111; //Apaga display.
delay_us(scan);
output_b(seg[dig2]);
cont.data=0b1011;//4
delay_us(scan);
cont.data=0b1111; //Apaga display.
delay_us(scan);
output_b(seg[dig1]);
cont.data=0b0111;//8
delay_us(scan);
cont.data=0b1111; //Apaga display.
delay_us(scan);;
} |
|
|
 |
dyeatman
Joined: 06 Sep 2003 Posts: 1933 Location: Norman, OK
|
|
Posted: Sat May 26, 2012 8:15 am |
|
|
Thank you ze, I think this can be helpful to some of the upcoming "newbies".
However, a couple of suggestions:
1. Add comments at the start of the program with a description of how the
PICS are connected and any other useful info.
2. Clean up and post in the Code Library for everyone.
These types of contributions are a help to all. Thanks for your efforts! _________________ Google and Forum Search are some of your best tools!!!! |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|