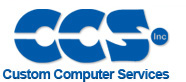 |
 |
View previous topic :: View next topic |
Author |
Message |
david90
Joined: 25 Feb 2006 Posts: 23
|
Software Uart and External Interrupt issue |
Posted: Sun Feb 20, 2011 4:48 pm |
|
|
Code: | //tested code with dev board.
#include <12F683.h>
#device adc=8
#FUSES NOWDT //No Watch Dog Timer
#FUSES INTRC //Internal RC Osc
#FUSES NOCPD //No EE protection
#FUSES NOPROTECT //Code not protected from reading
#FUSES NOMCLR //Master Clear pin used for I/O
#FUSES NOPUT //No Power Up Timer
#FUSES BROWNOUT //Reset when brownout detected
#FUSES IESO //Internal External Switch Over mode enabled
#FUSES FCMEN //Fail-safe clock monitor enabled
#use delay(clock=8000000)
#use rs232(baud=2400,parity=N,xmit=PIN_A1,rcv=PIN_A2,bits=8,errors)
int buffer[10];
int i=0;
int open_detected=0;
int close_detected=0;
void main()
{
setup_adc_ports(NO_ANALOGS|VSS_VDD);
setup_adc(ADC_OFF);
setup_timer_1(T1_DISABLED);
setup_timer_2(T2_DISABLED,0,1);
setup_comparator(NC_NC);
setup_vref(FALSE);
setup_oscillator(OSC_8MHZ);
enable_interrupts(INT_EXT);
ext_int_edge( H_TO_L );
enable_interrupts(GLOBAL); //enable interrupt
while(1)
{
output_high(PIN_A5);
delay_ms(100);
output_low(PIN_A5);
delay_ms(100);
if (open_detected==1)
{
output_high(PIN_A0);
delay_ms(100);
output_low(PIN_A0);
delay_ms(100);
output_high(PIN_A0);
delay_ms(100);
output_low(PIN_A0);
delay_ms(100);
open_detected=0;
}
if (close_detected==1)
{
output_high(PIN_A0);
delay_ms(100);
output_low(PIN_A0);
delay_ms(100);
close_detected=0;
}
}
}
#INT_EXT //use external pin int to avoid polling for serial data. Software UART does not have INT.
void INT_EXT_handler() {
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
buffer[0]=getc();
if ((buffer[0]==0x57 && buffer[1]==0xA9) || (buffer[0]==0x5F && buffer[1]==0xA1))
{
open_detected=1;
}
if (buffer[0]==0xDF && buffer[1]==0x21)
{
close_detected=1;
}
}
|
Whenever I power on my PIC, it immediately goes into the INT_EXT ISR and get stuck there until I send serial data to the PIC. I know for a fact that when I power up my pic, the pic doesn't even go to my infinite while loop but instead goes directly to the ISR. Why does this happen? There might be a chance that this happens because of my dev board. |
|
 |
temtronic
Joined: 01 Jul 2010 Posts: 9221 Location: Greensville,Ontario
|
|
Posted: Sun Feb 20, 2011 4:57 pm |
|
|
You might want to enable the PowerUpTimer ( PUT) as well as and a small delay in Main before the interrupts are enabled.
Depending on the board layout, power supply specs, etc. you might already have an interrupt from something 'glitchy'. |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Mon Feb 21, 2011 12:36 am |
|
|
Quote: |
Whenever I power on my PIC, it immediately goes into the INT_EXT ISR
|
Read this thread:
http://www.ccsinfo.com/forum/viewtopic.php?t=43138
Quote: |
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
shift_left(buffer,10,0);
|
Why do this ? Just use a pointer or an array index.
If you change this fuse to INTRC_IO then you can also use PIN_A4
as an i/o pin. |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|