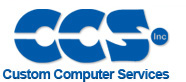 |
 |
View previous topic :: View next topic |
Author |
Message |
Ttelmah
Joined: 11 Mar 2010 Posts: 19499
|
|
Posted: Fri Apr 09, 2010 2:33 pm |
|
|
Obvious question though. What have you got connected to Vo.
If the voltage here is wrong, you could get two solid lines on the display.
Best Wishes |
|
 |
fushion3
Joined: 28 Sep 2006 Posts: 24
|
|
Posted: Mon Apr 12, 2010 9:07 am |
|
|
First I will address whats on Vo, it has a 5K pot to adj the contrast to LCD.
Here's the code I copied. Note the flex code has "B" in it because I made 2 versions. This code just has line 2 & line 4 solid on the LCD. I also change the clock from 4 to 20 MHz, no difference.
Code: |
#include <16F877.H>
#fuses XT, NOWDT, NOPROTECT, BROWNOUT, PUT, NOLVP
#use delay(clock = 4000000)
#include <Flex_LCD420B.c>
//===================================
void main()
{
int8 i;
int8 b1, b2, b3, b4;
// The lcd_init() function should always be called once,
// near the start of your program.
lcd_init();
// Clear the LCD.
printf(lcd_putc, "\f");
delay_ms(500);
while(1)
{
// Test the clear screen and newline commands.
// Also test that we can write to all 4 lines.
printf(lcd_putc, "\fThis is the 1st line");
printf(lcd_putc, "\nNext is the 2nd line");
printf(lcd_putc, "\nThis is the 3rd line");
printf(lcd_putc, "\nFinally the 4th line");
delay_ms(3000);
// Test some additional characters.
printf(lcd_putc, "\fABCDEFGHIJKLMNOPQRST");
printf(lcd_putc, "\nabcdefghijklmnopqrst");
printf(lcd_putc, "\n12345678901234567890");
printf(lcd_putc, "\n!@#$^&*(){}[]:;<>?/=");
delay_ms(3000);
// Clear the LCD.
printf(lcd_putc, "\f");
delay_ms(500);
// Test that lcd_gotoxy() works. Go to each of
// the four corners and put a number in each one,
// in a clockwise direction, starting with the upper
// left corner.
lcd_gotoxy(4, 2);
printf(lcd_putc, "Put a number in");
lcd_gotoxy(4, 3);
printf(lcd_putc, "each corner.");
lcd_gotoxy(1, 1);
printf(lcd_putc, "1");
lcd_gotoxy(20, 1);
printf(lcd_putc, "2");
lcd_gotoxy(20, 4);
printf(lcd_putc, "3");
lcd_gotoxy(1, 4);
printf(lcd_putc, "4");
delay_ms(3000);
// Read the character that was written in each corner
// of the LCD and display it. This tests the lcd_getc()
// function.
// The following test can only be done if we can read
// from the LCD. If the RW pin is not used, then the
// LCD is in write-only mode, and we can't do this test.
// The #ifdef statement will prevent the code from
// being compiled, in that case.
#ifdef USE_RW_PIN
// Test if lcd_getc() can read
// a byte from each corner.
b1 = lcd_getc(1,1);
b2 = lcd_getc(20,1);
b3 = lcd_getc(20,4);
b4 = lcd_getc(1,4);
lcd_gotoxy(1, 1);
printf(lcd_putc, "\fRead these bytes\n");
printf(lcd_putc, "from the 4 corners:\n\n");
printf(lcd_putc, " %c %c %c %c", b1, b2, b3, b4);
delay_ms(3000);
#endif
// Type some characters and backspace over them.
printf(lcd_putc, "\fType characters and\n");
printf(lcd_putc, "backspace over them.");
delay_ms(2000);
// Go to end of 2nd line.
lcd_gotoxy(20, 2);
// Backspace over 2nd line.
for(i = 0; i < 20; i++)
{
printf(lcd_putc," \b\b");
delay_ms(150);
}
// Go to end of first line.
lcd_gotoxy(20, 1);
// Backspace over first line.
for(i = 0; i < 20; i++)
{
printf(lcd_putc," \b\b");
delay_ms(150);
}
}
}
|
This code blinks random address on each of the 4 lines:
Code: |
#include <16F877A.h>
#device ICD=TRUE
#device ADC=10
#fuses HS,NOWDT,NOPROTECT,NOLVP
#use delay(clock=4000000) // 16Mhz Crystal is Used
#use rs232(baud=9600, parity=N, xmit=PIN_C6, rcv=PIN_C7, bits=8) // RS232 Configuration
#include <flex_lcd420.c>
#include <stdlib.h>
void main()
{
lcd_init();
while(1)
{
output_high(PIN_D1);
delay_ms(1000);
output_low(PIN_D1);
printf(lcd_putc, "Hello World.");
delay_ms(1000);
}
} |
|
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Mon Apr 12, 2010 12:34 pm |
|
|
Post a list of the pin connections between your PIC and the LCD.
Don't post the signal names. Just post, pin x on the PIC goes to pin y
on the LCD. (for all connections).
Also what is voltage that you have set on the Vo pin of the LCD,
with the trimpot ? |
|
 |
fushion3
Joined: 28 Sep 2006 Posts: 24
|
|
Posted: Mon Apr 12, 2010 1:46 pm |
|
|
I'm using a PIC 16F877A TQFP package;
LCD pin 1 (Vss) ground
LCD pin 2 (Vdd) 5V
LCD pin 3 (V0) has 0.50 volts
LCD pin 4 (RS) goes to PIC pin 40 (D2)
LCD pin 5 (R/W) goes to PIC pin 41 (D3)
LCD pin 6 (E) goes to PIC pin 39 (D1)
LCD pin 11 (DB4) goes to PIC pin 2 (D4)
LCD pin 12 (DB5) goes to PIC pin 3 (D5)
LCD pin 13 (DB6) goes to PIC pin 4 (D6)
LCD pin 14 (DB7) goes to PIC pin 5 (D7) |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Mon Apr 12, 2010 2:00 pm |
|
|
Your connections look OK. Your pin #define statements in the lcd
driver file should look like this:
Code: |
#define LCD_DB4 PIN_D4
#define LCD_DB5 PIN_D5
#define LCD_DB6 PIN_D6
#define LCD_DB7 PIN_D7
#define LCD_RS PIN_D2
#define LCD_RW PIN_D3
#define LCD_E PIN_D1
|
Quote: |
#use delay(clock=4000000) // 16Mhz Crystal is Used
|
Your comment says you're using a 16 MHz crystal, but you have the
#use delay set to 4 MHz. What crystal are you using ?
Quote: |
output_high(PIN_D1);
delay_ms(1000);
output_low(PIN_D1);
|
You have pin D1 assigned to the "E" pin on the LCD, but here you're
toggling it, as if it's a general purpose i/o pin.
Are you trying to Single-Step through this code? I would just let it run.
Quote: | I'm using a PIC 16F877A TQFP package; |
What is the Vdd voltage on this PIC ? |
|
 |
fushion3
Joined: 28 Sep 2006 Posts: 24
|
|
Posted: Tue Apr 13, 2010 8:23 am |
|
|
Yes, I tried different clocks to see if timing was an issue, but I have a 20MHz crystal in my setup.
Again, the code was copied and pasted into the compiler from this site, so comments are what they are, I just tweaked here and there to get things to compile and show what was changed if it needed to go back to original code.
The PIC is a 5V Vdd.
All I wanted is to test this LCD by seeing something simple like "hello world" on each line to make sure it works before I stick it on the 18F452 platform. I would really like to have the PIC read from the ADC's (done this before with great success) and display the readings on the LCD. |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Tue Apr 13, 2010 9:46 am |
|
|
Quote: |
I have a 20MHz crystal in my setup.
|
The #use delay() value must match the oscillator frequency.
Set it to this and leave it that way:
Code: |
#use delay(clock=20000000)
|
|
|
 |
fushion3
Joined: 28 Sep 2006 Posts: 24
|
|
Posted: Tue Apr 13, 2010 10:49 am |
|
|
I made the changes you suggested and here is what it looks like now, but still just blink random address in LCD.
Code: |
#include <16F877A.h>
//#device ICD=TRUE
#device ADC=10
#fuses HS,NOWDT,NOPROTECT,NOLVP
#use delay(clock=20000000) // 20Mhz Crystal is Used
#use rs232(baud=9600, parity=N, xmit=PIN_C6, rcv=PIN_C7, bits=8) // RS232 Configuration
#include <flex_lcd420.c>
#include <stdlib.h>
void main()
{
lcd_init();
while(1)
{
//output_high(PIN_D1);
//delay_ms(1000);
//output_low(PIN_D1);
printf(lcd_putc, "Hello World.");
delay_ms(1000);
}
} |
|
|
 |
MikeP
Joined: 07 Sep 2003 Posts: 49
|
|
Posted: Tue Apr 13, 2010 5:51 pm |
|
|
Code: | #include <16F877A.h>
//#device ICD=TRUE
#device ADC=10
#fuses HS,NOWDT,NOPROTECT,NOLVP
#use delay(clock=20000000) // 20Mhz Crystal is Used
#use rs232(baud=9600, parity=N, xmit=PIN_C6, rcv=PIN_C7, bits=8) // RS232 Configuration
#include <flex_lcd420.c>
#include <stdlib.h>
void main()
{
lcd_init();
printf(lcd_putc, "Hello World.");
while(TRUE){}; //keep PIC from going to sleep
} |
Since you are not resetting the display's address each time in that while loop I think the display is getting confused. |
|
 |
fushion3
Joined: 28 Sep 2006 Posts: 24
|
|
Posted: Wed Apr 14, 2010 10:26 am |
|
|
MikeP,
I tried your code and saw the only difference is how we write the while loop that basically does the same thing.
I have good news in that I use an USB interface to flash the HEX file unto the PIC and every now & then the code worked, but as soon as I went to my power supply board it just illuminated lines 2 & 4. I found out that the socket which holds the PIC TQFP package was missing Vdd on pin 28, thus not allow the PIC to run the code only allowing it to be flashed. With everything powered all four lines work great and thanks to all whom were patient enough to offer help, CHEERS! |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|