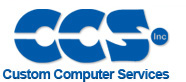 |
 |
View previous topic :: View next topic |
Author |
Message |
PaulW
Joined: 12 Mar 2010 Posts: 3
|
receiving garbage from SPI MISO |
Posted: Wed Mar 17, 2010 1:46 pm |
|
|
CCS PCH C Compiler, Version 4.011, 32099
PIC18F6722
MCP23S17 http://ww1.microchip.com/downloads/en/DeviceDoc/21952b.pdf
Problem:
I'm trouble shooting a problem with SPI port 2 on PIC.
Conditions:
Existing project has trouble reading SPI port 2. It's using bit banging. RX signals look good, but Data isn't reliable.
What I've tried:
Wrote small project (see below) to test SPI 2. I'm Using CCS canned SPI access functions. It compiles and runs. My CS, CLK, Data out looks great. Slave Out data is at correct sequence, but logic levels look bizarre.
Both of these projects are running on the same board.
What I'm trying to accomplish: Write a small test program that will simple read from GPIO A and GPIO B, that I can use to verify that: 1. Hardware works. 2. Figure out what is wrong in existing Project.
Does this code look right?
Code: |
#include <18F6722.h>
#fuses HS, NOWDT, NOPROTECT, BROWNOUT, PUT, NOLVP
#use delay(clock=20000000)
#use rs232(baud=9600, xmit=PIN_C6, rcv=PIN_C7, ERRORS)
#define GPIO_EXTENDER_CS PIN_D3
// SPI modes
#define SPI_MODE_0 (SPI_L_TO_H | SPI_XMIT_L_TO_H)
#define SPI_MODE_1 (SPI_L_TO_H)
#define SPI_MODE_2 (SPI_H_TO_L)
#define SPI_MODE_3 (SPI_H_TO_L | SPI_XMIT_L_TO_H)
//----------------------------------
void GPIO_Write_Register(int8 reg, int8 data)
{
int16 temp;
output_low(GPIO_EXTENDER_CS);
temp = ((reg & 0x3F) << 1) | 0x40; // write to address 0x42 - (for debugger watch)
spi_write2(temp); // set up address
spi_write2(data); // send data
output_high(GPIO_EXTENDER_CS);
}
//---------------------------------
int8 GPIO_Read_Register(int8 reg)
{
int8 data;
output_low(GPIO_EXTENDER_CS);
spi_write2((((reg & 0x3F) << 1) | 0x01) | 0x40); // send read address to 0x42
data = spi_read2(0); // read
output_high(GPIO_EXTENDER_CS);
return(data);
}
//===============================
void main()
{
int8 result;
int8 Xdata, Ydata, Zdata;
output_high(GPIO_EXTENDER_CS);
delay_ms(10); // Minimum 1 ms required after power-up.
setup_spi2(SPI_MASTER | SPI_MODE_0 | SPI_CLK_DIV_16); // 1.25 MHz sclk
// Acc_Write_Register(0x1, 0x12);
delay_ms(30); // Minimum 20 ms required after exiting standby
for(;;)
{
GPIO_Write_Register(0x1, 0x12); // set register pointer to 0x12
Xdata = GPIO_Read_Register(0x01); // read from register 0x12
Ydata = GPIO_Read_Register(0x01); // read from register 0x13
delay_ms(500);
}
}
|
I have a screen shot of the data out, if anyone interested. Thanks. |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Wed Mar 17, 2010 2:04 pm |
|
|
Quote: | Slave Out data is at correct sequence, but logic levels look bizarre.
Both of these projects are running on the same board.
|
If the logic levels look weird, then look for two output pins that are
connected together (or shorted), and are fighting each other. |
|
 |
ckielstra
Joined: 18 Mar 2004 Posts: 3680 Location: The Netherlands
|
|
Posted: Wed Mar 17, 2010 3:05 pm |
|
|
Besides the likely electrical problems, your compiler version is highly unstable. It has been mentioned many times on this forum: don't use any v4.0xx compiler older than v4.070
These were alpha quality releases of the new compiler and should never have been released without a huge neon warning message.
Save everybody, yourself included, a lot of time by upgrading to a new compiler or downgrade to the stable v3.249 that was available at the time of your v4.011 download. |
|
 |
PaulW
Joined: 12 Mar 2010 Posts: 3
|
|
Posted: Wed Mar 17, 2010 3:22 pm |
|
|
That's what it looks like to me too, but I can find no cross talk. I've tripled checked everything. No shorted pins on the chip in place. With Everything connected, there is no shorted pins either.
The chip seems to be responding to requests, but it's wacky. It's like cap limited, (or something). Slave Out goes high, then writes, but peters out, and slews down to zero again.
My Problem is though:
The Chip output levels look good when bit banged. ???
The Chip output looks really strange with the above code. ???
The only difference is Software. ???
It's the same board. Same connections. And I tried it on two other boards too. They all do the same thing, and behave differently with the two softwares installed.
Does the above code look reasonable?
I'm thinking It's probably the chip setup somehow.
Thank you. |
|
 |
PaulW
Joined: 12 Mar 2010 Posts: 3
|
|
Posted: Wed Mar 17, 2010 3:23 pm |
|
|
@ckielstra, Thanks. I do have that version and will try it too. |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Wed Mar 17, 2010 3:34 pm |
|
|
Post a list of the connections between the PIC and the MCP23S17.
Look at the physical wires (or tracks) on the board and post the
pin-to-pin list of connections, taken by direct visual observation.
Don't post the signal names. Post the pins. |
|
 |
a3ka2000
Joined: 26 Feb 2010 Posts: 11
|
|
Posted: Thu Mar 18, 2010 1:53 am |
|
|
Hi, just a question, for some PIC model that does not have SPI_XMIT_L_TO_H defined in the header file, does that mean that it's not available? How to define SPI Mode0 then? |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|