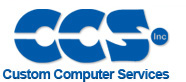 |
 |
View previous topic :: View next topic |
Author |
Message |
Guest
|
functions |
Posted: Tue Mar 02, 2010 3:40 am |
|
|
hi there,
i have a code which get data from a rs232 gps devices in side it's own function, this all works fine.
Code: |
void get_gps(){
do{
cget = fgetc(GPS);
fprintf(PC,"%s\n",cget);
}}
|
but i have another code in the main, which has a constant while loop:
Code: |
main() {
while (True)
{
(if statements); - this is the other code
void get_gps();
delay_ms(500);
}
} |
only thing that works is the void get_gps(); the if statements in the while loop doesnt work.
i there a way of making both work with out using a interrupt?
thanks
Jonny b |
|
 |
Eddy71ua
Joined: 23 Sep 2009 Posts: 55 Location: Ukraine
|
Re: functions |
Posted: Tue Mar 02, 2010 3:47 am |
|
|
Anonymous wrote: | hi there,
Code: |
main() {
while (True)
{
(if statements); - this is the other code
get_gps();
delay_ms(500);
}
} |
|
Try this code  |
|
 |
Wayne_
Joined: 10 Oct 2007 Posts: 681
|
|
Posted: Tue Mar 02, 2010 4:00 am |
|
|
Code: |
void get_gps(){
do{
cget = fgetc(GPS);
fprintf(PC,"%s\n",cget);
}
}
|
This also has an infinite loop in it. Once you enter this routine it WILL NOT exit. Actually it will probably not compile without the while statement!
You can fix this several ways and it all depends on what else your code is doing.
If you know how much data you will receive :-
Code: |
void get_gps(){
int i = 10; // Receive 10 chars
for (; i > 0; i--)
{
cget = fgetc(GPS);
fprintf(PC,"%s\n",cget);
}
}
|
I would not use this, if you miss a char or the number of chars is different to what you expect your code will either wait indefinitely or get out of sync.
If you know what the termination char is for your data (usually '\r' or '\n') for modems then keep reading until you get this.
Code: |
void get_gps(){
do{
cget = fgetc(GPS);
fprintf(PC,"%s\n",cget);
} while (cget != '\r');
}
|
or just use gets instead:-
Code: |
void get_gps(){
cget = fgets(GPS);
fprintf(PC,"%s\n",cget);
}
|
What is cget defined as ?
Your printf is expecting a string but you use fgetc to read a single char!
Last edited by Wayne_ on Tue Mar 02, 2010 4:02 am; edited 1 time in total |
|
 |
Ttelmah Guest
|
|
Posted: Tue Mar 02, 2010 4:02 am |
|
|
A number of separate problems:
First, if you do get to the outside loop, because of the long delay (500mSec), you _will_ miss RS232 data, and possibly hang the UART....
Second, the get_gps function, will sit _waiting_ for data to arrive from the GPS. While doing so, nothing in the external loop will be executed...
Now, whether this can be done without interrupts, depends on how long the 'if' statements will take. If the total is more than one character time, you will risk missing serial data. But the basic approach would need to be something like:
Code: |
#define LOOPSPERTEST (255)
main() {
int8 loopcount=LOOPSPERTEST;
while (True) {
if (loopcount) --loopcount;
else {
loopcount=LOOPSPERTEST;
//other code here;
}
get_gps();
}
}
//You adjust 'LOOPSPERTEST' to adjust how frequently the 'other code'
//is executed
void get_gps(void) {
if (kbhit(GPS)) {
cget = fgetc(GPS); //Only read the GPS if a character is waiting
fprintf(PC,"%c",cget);
}
}
|
There is another problem in your code, which actually suggests that 'get_gps', is _not_ working. 'cget', is a character, not a string. When you print it as a string, the code will print everything from memory, till it happens to hit a '\0' character.
The void declaration in calling the funstion from main, is redundant.
The GPS stream, needs to be using a hardware UART, for this to work.
Best Wishes |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|