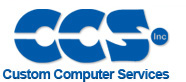 |
 |
View previous topic :: View next topic |
Author |
Message |
johnnyD Guest
|
USB HID transmit problem |
Posted: Tue Sep 23, 2008 2:52 pm |
|
|
Hello ya'll!
I have modified the ex_usb_hid example. I can receive up to 64 bytes from a PC with no problem. Where I am getting stuck is trying to transmit x number of bytes to the PC. The problem is in the TX report count in the HID descriptor.
It works great if I set the TX report count to 5 in my HID descriptor and output 5 bytes, then the PC is able to read the data.
if I set the TX report count say to 10 and send out 9 bytes the PC doesn't read the data
is there any way to be able to transmit x number bytes that the PC can read?
Code: |
#include <18F2550.h>
#device PASS_STRINGS=IN_RAM
#fuses HSPLL,NOWDT,NOPROTECT,NOLVP,NODEBUG,USBDIV,PLL5,CPUDIV1,VREGEN
#use delay(clock=48000000)
#define USB_HID_DEVICE TRUE
#define USB_EP1_TX_ENABLE USB_ENABLE_INTERRUPT
#define USB_EP1_TX_SIZE 64
#define USB_EP1_RX_ENABLE USB_ENABLE_INTERRUPT
#define USB_EP1_RX_SIZE 64
#include <pic18_usb.h>
#include <usb_desc_hid.h>
#include <usb.c>
int in_data[64]={0};
int out_data[5]={0x41,0x42,0x43,0x44,0x45};
void main()
{
int1 ready=false;
usb_init();
while (TRUE)
{
usb_task();
if (usb_enumerated())
{
usb_put_packet(1, out_data, 5, USB_DTS_TOGGLE);
//while(usb_kbhit(1))
//{
// usb_get_packet(1,in_data,64);
//ready=true;
//}
// if(ready)
// {
// printf("%s\r",in_data);
// ready=false;
// }
}
}
}
|
Here is my descriptor code
Code: |
const char USB_CLASS_SPECIFIC_DESC[] = {
6, 0, 255, // Usage Page = Vendor Defined
9, 1, // Usage = IO device
0xa1, 1, // Collection = Application
0x19, 1, // Usage minimum
0x29, 8, // Usage maximum
0x15, 0x80, // Logical minimum (-128)
0x25, 0x7F, // Logical maximum (127)
0x75, 8, // Report size = 8 (bits)
0x95, 5, // TX report count
0x81, 2, // Input (Data, Var, Abs)
0x19, 1, // Usage minimum
0x29, 8, // Usage maximum
0x75, 8, // Report size = 8 (bits)
0x95, 63, // RX report count = 64 bits
0x91, 2, // Output (Data, Var, Abs)
0xC0 // End Collection
};
|
|
|
 |
Dave_Mustaine Guest
|
USB HID transmit problem |
Posted: Tue Sep 23, 2008 4:06 pm |
|
|
Give this a try: The key is your data lengths must all match. The remainder of your packet will be all zeros but you can always parse them!
Good Luck
Code: |
#include <18F2550.h>
#device PASS_STRINGS=IN_RAM
#fuses HSPLL,NOWDT,NOPROTECT,NOLVP,NODEBUG,USBDIV,PLL5,CPUDIV1,VREGEN
#use delay(clock=48000000)
#define USB_HID_DEVICE TRUE
#define USB_EP1_TX_ENABLE USB_ENABLE_INTERRUPT
#define USB_EP1_TX_SIZE 32
#define USB_EP1_RX_ENABLE USB_ENABLE_INTERRUPT
#define USB_EP1_RX_SIZE 32
#include <pic18_usb.h>
#include <usb_desc_hid.h>
#include <usb.c>
#include <string.h>
int in_data[32]={0}; // Set array to zero
int out_data[32]={0}; // Set array to zero
void main()
{
int1 ready=false;
strcpy(out_data,"HELLO THERE!");
usb_init();
while (TRUE)
{
usb_task();
if (usb_enumerated())
{
while(usb_kbhit(1))
{
usb_get_packet(1,in_data,31);
ready=true;
}
if(ready)
{
printf("%s\r",in_data);
ready=false;
usb_put_packet(1, out_data, 31, USB_DTS_TOGGLE);
}
}
}
}
|
Now set your descriptor for the following
Code: |
const char USB_CLASS_SPECIFIC_DESC[] = {
6, 0, 255, // Usage Page = Vendor Defined
9, 1, // Usage = IO device
0xa1, 1, // Collection = Application
0x19, 1, // Usage minimum
0x29, 8, // Usage maximum
0x15, 0x80, // Logical minimum (-128)
0x25, 0x7F, // Logical maximum (127)
0x75, 8, // Report size = 8 (bits)
0x95, 31, // TX report count = 32 bits
0x81, 2, // Input (Data, Var, Abs)
0x19, 1, // Usage minimum
0x29, 8, // Usage maximum
0x75, 8, // Report size = 8 (bits)
0x95, 31, // RX report count = 32 bits
0x91, 2, // Output (Data, Var, Abs)
0xC0 // End Collection
};
|
|
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|