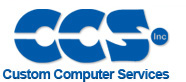 |
 |
View previous topic :: View next topic |
Author |
Message |
piripic
Joined: 15 Jan 2008 Posts: 25
|
help, how to cast signed variable ? |
Posted: Tue Jul 01, 2008 2:58 am |
|
|
Hi, I am in trouble to understanding how to make to work this example. Can anyone explain to me how to cast (if possible) signed variables ? Thanks
Claudio
Code: | #include <16F877.H>
#fuses XT, NOWDT, NOPROTECT, BROWNOUT, PUT, NOLVP
#use delay(clock = 8000000)
#use rs232(baud=9600, xmit=PIN_C6, rcv=PIN_C7, ERRORS)
//------------------------------------------------------------------------------
// Return the temperature in ° centigrade
signed int8 TempRead(int8 valore)
{
// Temp [°C] = -40 + (68.4 - TEMP READBACK) × 9.32
signed int32 temperat, temper32;
int16 temp_rd;
// To avoid floating point numbers I multiply all values by 100
temp_rd = ((int8)valore & 0x7F);
temper32 = 6840 - temp_rd * 100;
temperat = -4000 + temper32 * 932;
temperat /= 100;
#asm
BSF &(int8)temperat,7 // Set bit 7 of temperat LSByte
BTFSS &(int8)temperat+3,7 // Skip if MSB (bit 31 of temperat) = 1
BCF &(int8)temperat,7 // Clear bit 7 of temperat LSByte
#endasm
return (signed int8)temperat;
}
//======================================
void main()
{
signed int8 mytemp;
int8 value;
while(1) {
while(!kbhit()) ;
value = getc();
mytemp = TempRead(value);
printf("Value = %u \n\rTemperature = %d \n\r", value, mytemp);
}
} |
|
|
 |
Ttelmah Guest
|
|
Posted: Tue Jul 01, 2008 5:16 am |
|
|
I don't think you mean 'cast', possibly just 'output'?.
'casting', is forcing the conversion of a variable into another type. So (for example), you can take a sum like:
Code: |
int8 a,b;
int16 val;
a=100;
b=100;
val = (int16)a*b;
|
Which forces 'a' to be converted into an int16 type, before the arithmetic is performed (and thereby forces 'int16' arithmetic).
I suspect what you are asking is how to output a 'long' type?. The answer is 'L'.
So:
Code: |
printf("%Ld/n/r",val);
|
Switches the output to support the 'long'.
'd', and 'x', _default_ to assuming the value is 'signed'. If you want to use unsigned values, you use 'u' (which you are already doing).
Best Wishes |
|
 |
piripic
Joined: 15 Jan 2008 Posts: 25
|
|
Posted: Tue Jul 01, 2008 8:40 am |
|
|
Ttelmah, thanks. I think I have found the mistake that I did.
For "cast" I meant to say... to pass a signed int32 to a signed int8. I believe that using the three assembly instructions all work good... I 'shift' the sign bit.
The example below seems to work... obviously the range of 'temperat' does not exceed the -128 +127...
Code: | #include <16F877.H>
#fuses XT, NOWDT, NOPROTECT, BROWNOUT, PUT, NOLVP
#use delay(clock = 8000000)
#use rs232(baud=9600, xmit=PIN_C6, rcv=PIN_C7, ERRORS)
//------------------------------------------------------------------------------
// Return the temperature in ° centigrade
signed int8 TempRead(int8 valore)
{
// Temp [°C] = -40 + (68.4 - TEMP READBACK) × 9.32
signed int32 temperat, temper32;
int16 temp_rd;
// To avoid floating point numbers I multiply values by 100
temp_rd = ((int8)valore & 0x7F);
temper32 = 6840 - (signed int16)temp_rd * 100;
temperat = (temper32 * 932) / 10000;
temperat += -40;
#asm // Now I 'shift' the sign bit
BSF &(int8)temperat,7 // Set bit 7 of temperat (least significant byte)
BTFSS &(int8)temperat+3,7 // Skip if MSB (bit 31 of temperat) = 1
BCF &(int8)temperat,7 // Clear bit 7 of temperat (least significant byte)
#endasm
return (signed int8)temperat;
}
//======================================
void main()
{
signed int8 mytemp;
int8 value;
while(1) {
while(!kbhit()) ;
value = getc();
mytemp = TempRead(value);
printf("Value = %u \n\rTemperature = %d \n\r", value, mytemp);
}
} |
Thanks again,
Claudio |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|