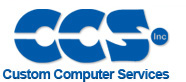 |
 |
View previous topic :: View next topic |
Author |
Message |
avjarun
Joined: 28 Mar 2021 Posts: 12
|
Lora Module Communication |
Posted: Tue Sep 24, 2024 10:57 pm |
|
|
Hi All.
I am trying to establish a communication with Lora SX1278 module with SPI. I am using 18F18323 (Internal Crystal). I have a problem with the below SPI communication functions. It doesn't seem to be communicating and is struck in the while loop. I am unable to trace the mistake that I am doing.
Connections as follows and double checked the same. PIC operates @ 3.3V
PIC16F18323 SX1278
------------ ------------
VCC -------------- VCC
GND -------------- GND
PIN_C0 ----------- NSS
PIN_C1 ----------- RESET
PIN_C5 ----------- MOSI (DO)
PIN_C4 ----------- MISO (DI)
PIN_C3 ----------- SCK
PIN_C2 ----------- LED
Pls advice on the above.
Code: |
#include <16F18323.h>
#device ADC=10
#fuses NOWDT, NOPROTECT, BROWNOUT, PUT
#use delay(internal=8MHz)
#use spi(MASTER, DO=PIN_C5, DI=PIN_C4, CLK=PIN_C3, MODE=0, BITS=8, STREAM=SPI_STREAM)
#define NSS_PIN PIN_C0 // SX1278 NSS (chip select) pin
#define RESET_PIN PIN_C1 // SX1278 Reset pin
#define LED_PIN PIN_C2 // LED pin to glow on successful reception
// SX1278 Register Addresses
#define REG_OP_MODE 0x01
#define REG_VERSION 0x42 // Version register for SPI test
// Function to select SX1278 by pulling NSS low
void SX1278_Select() {
output_low(NSS_PIN);
delay_us(10);
}
// Function to deselect SX1278 by pulling NSS high
void SX1278_Deselect() {
delay_us(10);
output_high(NSS_PIN);
}
// Function to reset SX1278
void SX1278_Reset() {
output_low(RESET_PIN);
delay_ms(200); // Increased reset delay
output_high(RESET_PIN);
delay_ms(200);
}
// SPI Write to SX1278 register
void SX1278_Write_Register(int8 addr, int8 value) {
SX1278_Select();
spi_xfer(SPI_STREAM, addr | 0x80); // Write mode (MSB is 1)
spi_xfer(SPI_STREAM, value);
SX1278_Deselect();
}
// SPI Read from SX1278 register
int8 SX1278_Read_Register(int8 addr) {
int8 value;
SX1278_Select();
spi_xfer(SPI_STREAM, addr & 0x7F); // Read mode (MSB is 0)
value = spi_xfer(SPI_STREAM, 0x00);
SX1278_Deselect();
return value;
}
void main() {
setup_comparator(NC_NC_NC_NC); // Disable all comparators
// Setup pins: Set C0 (NSS), C1 (Reset), C2 (LED), C3 (SCK), C4 (MISO), C5 (MOSI)
set_tris_c(0b00110000); // Configure TRISC: SCK, MISO as input, others output
output_high(LED_PIN); // Turn on LED to indicate setup start
// Initialize SPI
setup_spi(SPI_MASTER | SPI_CLK_DIV_16);
// Reset SX1278
SX1278_Reset();
// SPI Communication Test: Read REG_VERSION (should return 0x12)
if (SX1278_Read_Register(REG_VERSION) == 0x12) {
output_low(LED_PIN); // Turn off LED if register read is successful
delay_ms(1000);
} else {
while(1) {
output_toggle(LED_PIN); // Blink LED to indicate SPI failure
delay_ms(100);
}
}
while(1)
{
output_high(LED_PIN);
delay_ms(1000);
}
}
|
|
|
 |
diode_blade
Joined: 18 Aug 2014 Posts: 55 Location: Sheffield, South Yorkshire
|
|
Posted: Wed Sep 25, 2024 1:27 am |
|
|
You have no EXIT condition for the 'WHILE' Loop so you will always be stuck in it.
Code: |
while(1 or true){
do something //always stuck here.
}
|
Now some code I wrote for a keypress subroutine check out the exit from the while
Code: |
Char calibration(void)
{
char keypress; // keypress value
keypress='0';
glcd_fillscreen(off);
glcd_update();
while(keypress!='E'){ //STAY IN WHILE LOOP UNITIL KEY 'E' IS PRESSED
keypress=kbd_getc(); //read keypad
glcd_pos(5,1);
printf(glcd_putc,"Calibration ");
glcd_pos(5,15);
printf(glcd_putc,"Press 2 for 2 Point");
glcd_pos(5,25);
printf(glcd_putc,"Press 5 for 5 Point");
glcd_pos(5,35);
printf(glcd_putc,"ESC to Exit");
glcd_update();
if(keypress=='2'){
two_pt flag=true; ///set flag for 2 point
five_pt=false;
} //end if
if(keypress=='5'){
five_pt flag =true; //set flag for 5 point
two_pt=false;
} //end if
} //end while loop if keypress == E pressed
glcd_fillscreen(off);
glcd_update();
return(keypress);
} //END of Subroutine
|
You need to be checking for an exit condition in your while loop. |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19495
|
|
Posted: Wed Sep 25, 2024 1:44 am |
|
|
What compiler version?.
Now some comments. You are mixing using two different ways of handling
the SPI. Historically there was setup_spi, and spi_read/write. This was
the old way that is only designed for chips with a fixed pin peripheral.
By default this will talk to the spa on pins RC0/RC1, which is the default
location. The second way is to use #use spy, and spy_xfer. Depending on
your compiler version (hence the question above), this can handle using
PPS to relocate the pins. However it should not be mixed with the setup_spi
commands. These are not compatible with one another (except in a few
very specific cases).
Even on compilers that can handle the PPS, it is honestly always safer
to just do it yourself.
So:
Code: |
//Change your SPI setup to:
include <16F18323.h>
#device ADC=10
#fuses NOWDT, NOPROTECT, BROWNOUT, PUT
#use delay(internal=8MHz)
#PIN_SELECT SDO1=PIN_C5
#PIN_SELECT SDI1=PIN_C4
#PIN_SELECT SCL1=PIN_C3
#use spi(MASTER, MODE=0, BITS=8, BAUD=125K, STREAM=SPI_STREAM)
|
Now I have set the baud to the slow rate you are using in your spi_setup.
Why so slow?.
Get rid of your spi_setup line.
This ensures the hardware SPI is actually setup and used.
Looking through your code, the reads and writes look correct to at
least read the version register.
Well done on leaving the times needed after setup, and your pin
mapping looks right. Also mode number is correct.
Only other thing I would do, is pause at the start. The chip takes
10mSec to wake after POR. Now PIC's are very quick at starting, and
may well start before the supply is up to the voltage this chip needs.
So I'd wait at the start for perhaps 15mSec before your wake up
sequence.
Presumably this is a pre-wired module, so we don't have to worry that
you have got the chips oscillator wired up correctly etc..  |
|
 |
temtronic
Joined: 01 Jul 2010 Posts: 9221 Location: Greensville,Ontario
|
|
Posted: Wed Sep 25, 2024 7:15 am |
|
|
also....
most LORA modules, well 'any' wifi comm chips need 100s of ma to transmit ! While low power in receive mode, check the datasheet for 'transmit power'.
If it uses ,say 150ms your power supply should be rated for say 500 ma. I use a x10 factor. Module needs 100, I use a 1,000ma (1 a) supply.
This is IN ADDITION to whatever current the PIC and parts require, worst case ( all LEDS on, transmitting, etc.) |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|