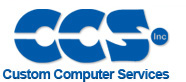 |
 |
View previous topic :: View next topic |
Author |
Message |
complex72
Joined: 10 May 2014 Posts: 11
|
TOUCH SLIDER |
Posted: Sat May 10, 2014 11:02 pm |
|
|
Hi I've been doing some work using the TOUCHPAD functions for 3 buttons and a slider, Ive got the buttons to work perfect but how can I get the slider (2 inverse triangles) to work with this functions? I've read the AN1250 pdf provided by microchip and they actually give a formula on how to calculate the position, but is there a way to do this with the compiler? thank you
#USE TOUCHPAD (RANGE=H,THRESHOLD=3,SCANTIME=32,PIN_B0='1',PIN_B1='2',PIN_B2='3')
void main(void)
{
char c;
enable_interrupts(GLOBAL);
while (TRUE)
{
if ( TOUCHPAD_HIT() )
c = TOUCHPAD_GETC(); //gets wich cap button was toched
switch ('c')
{
case '1':
output_high(pin_c0);
output_high(pin_a5);
output_low(pin_c3);
break;
case '2':
output_low(pin_c0);
output_high(pin_a5);
output_low(pin_c3);
break;
case '3':
output_low(pin_c0);
output_low(pin_a5);
output_high(pin_c3);
break;
}
}
} |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19496
|
|
Posted: Sun May 11, 2014 4:10 am |
|
|
Yes.
The key is to use the touchpad_state function, not the getc.
touchpad_state(1);
stores the values from the connected pads as int16 numbers directly into the array 'TOUCHDATA'. It's defined to have the size for the number of pins you use. Then TOUCHDATA[0] is the actual direct reading from the first pad etc..
You ignore the threshold etc., and just look at the values from the two pads you use for the slider, One will go up, and the other down, as the finger moves along the slider.
Add the pins you are using for the slider to your touchpad setup, giving them 'dummy' return values. Write your function that reads the keypad getc, to ignore these dummy values, and just read the numbers directly from the array, when they are seen (signal is above threshold).
Best Wishes |
|
 |
complex72
Joined: 10 May 2014 Posts: 11
|
|
Posted: Sun May 11, 2014 6:45 pm |
|
|
Ttelmah wrote: | Yes.
The key is to use the touchpad_state function, not the getc.
touchpad_state(1);
stores the values from the connected pads as int16 numbers directly into the array 'TOUCHDATA'. It's defined to have the size for the number of pins you use. Then TOUCHDATA[0] is the actual direct reading from the first pad etc..
You ignore the threshold etc., and just look at the values from the two pads you use for the slider, One will go up, and the other down, as the finger moves along the slider.
Add the pins you are using for the slider to your touchpad setup, giving them 'dummy' return values. Write your function that reads the keypad getc, to ignore these dummy values, and just read the numbers directly from the array, when they are seen (signal is above threshold).
Best Wishes |
---------------------------------------------------------------------
Thank you Ttelmah I'm trying to use the int16 array TOUCHDATA but the compiler marks as a undefine, so what I did was to define it as
unsigned int16 TOUCHDATA[];, after that I configured my serial port to debug and see what values are stored in the array when I touch pin_c5 cap input. (its the only one defined)
Code: |
for(i=0;i<=16;i++)
{
fprintf(PC,"%s%u%s%lu\n","TOUCHDATA[",i,"] =",TOUCHDATA[i]);
}
delay_ms(500);
}
|
as results I got the 16 values stored in the array, but I got values in almost all of them:
TOUCHDATA[0] =0
TOUCHDATA[1] =1
TOUCHDATA[2] =257
TOUCHDATA[3] =1
TOUCHDATA[4] =0
TOUCHDATA[5] =0
TOUCHDATA[6] =72
TOUCHDATA[7] =0
TOUCHDATA[8] =512
TOUCHDATA[9] =26112
TOUCHDATA[10] =1611
TOUCHDATA[11] =59598
TOUCHDATA[12] =30333
TOUCHDATA[13] =32329
TOUCHDATA[14] =54696
TOUCHDATA[15] =2059
TOUCHDATA[16] =32512
TOUCHDATA[0] =0
TOUCHDATA[1] =1
TOUCHDATA[2] =257
TOUCHDATA[3] =1
TOUCHDATA[4] =0
TOUCHDATA[5] =0
TOUCHDATA[6] =72
TOUCHDATA[7] =0
TOUCHDATA[8] =1024
TOUCHDATA[9] =26112
TOUCHDATA[10] =1611
TOUCHDATA[11] =59598
TOUCHDATA[12] =30333
TOUCHDATA[13] =32329
TOUCHDATA[14] =54696
TOUCHDATA[15] =2059
TOUCHDATA[16] =32512
I noticed that the position 8 was changed when I touched and released the button, does that mean that position 8 is the button I'm sensing? Could you give me a simple pseudocode example so i could work on it?
than you |
|
 |
complex72
Joined: 10 May 2014 Posts: 11
|
|
Posted: Sun May 11, 2014 10:05 pm |
|
|
Does someone know about this topic that could help me out? thank you |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19496
|
|
Posted: Mon May 12, 2014 9:03 am |
|
|
I 'played' with this quite a few versions ago, so things might well be different....
I defined the array to have the same number of entries as the number of pins supported by the CTMU, and the order in the array seemed to correspond to the ADC channel numbers.
I did a calibration pass before starting (touchpad_state(1)), delayed, and then tested 'if (touchpad_hit())', to tell if any pin was above it's threshold. Once I saw one was I triggered the touchpad_state(2), waited for long enough to ensure this had triggered, and read the array.
I actually had to give up on using the functions, because though they worked on another chip, they were not working correctly on the chip I wanted to use, so I went 'diy' on the functions. This was though quite a few compiler versions ago!...
Key was though that things didn't happen immediately. If you triggered the touchpad_state(2) function, you had to wait for the timer interrupt to trigger before things updated. |
|
 |
complex72
Joined: 10 May 2014 Posts: 11
|
|
Posted: Mon May 12, 2014 9:40 am |
|
|
Ttelmah wrote: | I 'played' with this quite a few versions ago, so things might well be different....
I defined the array to have the same number of entries as the number of pins supported by the CTMU, and the order in the array seemed to correspond to the ADC channel numbers.
I did a calibration pass before starting (touchpad_state(1)), delayed, and then tested 'if (touchpad_hit())', to tell if any pin was above it's threshold. Once I saw one was I triggered the touchpad_state(2), waited for long enough to ensure this had triggered, and read the array.
I actually had to give up on using the functions, because though they worked on another chip, they were not working correctly on the chip I wanted to use, so I went 'diy' on the functions. This was though quite a few compiler versions ago!...
Key was though that things didn't happen immediately. If you triggered the touchpad_state(2) function, you had to wait for the timer interrupt to trigger before things updated. |
-----------------------------------------------------------------------------------
Thanks once again Ttelmah, ok basically im using the PIC18F46K22 and it says it has 28 Cap Touch Channels so my defined array will be of that size right? something like unsigned int16 TOUCHDATA[28];
Before entering the while loop I could do the calibration touchpad_state(1), and then check the touchpad_hit in the loop, when detected trigger touchpad_state(2) giving enough delay so I could then check the array.
I think I got it just a pair of last questions.
Is the array created automatically going to load the TOUCHDATA reading values as int16s?
If I'm just sensing for instance one cap button (only that one is defined) is the array suppose to throw out that value and the rest are going to be 0?
thanks |
|
 |
complex72
Joined: 10 May 2014 Posts: 11
|
Got it working...! |
Posted: Wed May 14, 2014 3:45 pm |
|
|
I talked with CCSC technical support and got the answer, the TOUCHDATA array isn't working because it doesn't exist, they found the issue and are going to fix it in the next version of the compiler, the real name of the array is in fact CTMU_THRESHOLD.
The data for each touch pin is stored in the array as two bytes, the index of the array that the touch pin data is stored in is based on which analog pin it is. For example if your using pin B0 as a touch pin, which is AN12 (PIC18F46K22) the least significant byte is in CTMU_THRESHOLD[24] and the most significant byte is in CTMU_THRESHOLD[25]. As an example you could use the next table:
-----------------------------------------
-----------LSB MSB
AN0-------0-----1
AN1-------2-----3
AN2-------4-----5
AN3-------6-----7
AN4-------8-----9
AN5------10----11
.
.
.
.
.
AN12-----24----25
--------------------------------------------
you could then take both bytes and do a make16 to get the real value.
Thanks alot Ttelmah and CCS support staff for your replies.! |
|
 |
complex72
Joined: 10 May 2014 Posts: 11
|
Re: Got it working...! |
Posted: Wed May 14, 2014 4:06 pm |
|
|
complex72 wrote: | I talked with CCSC technical support and got the answer, the TOUCHDATA array isn't working because it doesn't exist, they found the issue and are going to fix it in the next version of the compiler, the real name of the array is in fact CTMU_THRESHOLD.
The data for each touch pin is stored in the array as two bytes, the index of the array that the touch pin data is stored in is based on which analog pin it is. For example if your using pin B0 as a touch pin, which is AN12 (PIC18F46K22) the least significant byte is in CTMU_THRESHOLD[24] and the most significant byte is in CTMU_THRESHOLD[25]. As an example you could use the next table:
-----------------------------------------
-----------LSB MSB
AN0-------0-----1
AN1-------2-----3
AN2-------4-----5
AN3-------6-----7
AN4-------8-----9
AN5------10----11
.
.
.
.
.
AN12-----24----25
--------------------------------------------
you could then take both bytes and do a make16 to get the real value.
Thanks alot Ttelmah and CCS support staff for your replies.! |
just got feedback from support and the final way of implementing this is as his own words is:
Quote: |
"I was mistaken earlier, the data in the array is only 8 bits wide. So AN0 is at index 0, AN1 is at index 1, AN2 is at index 2, ....., AN12 is at index 12"
|
Thanks hope this helps |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|