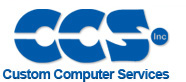 |
 |
View previous topic :: View next topic |
Author |
Message |
Guest
|
who have sorcecode of LCD in 8 bit mode. Please post me sour |
Posted: Mon Aug 16, 2004 8:21 pm |
|
|
who have sorcecode of LCD in 8 bit mode. Please post me sourcecode. because my sourcecode is not stable. it have 1 line. sometime it have 2 line. i use 16f877 and lcd 16*2 char. thank you for your help |
|
 |
Guest
|
|
Posted: Tue Aug 17, 2004 10:11 am |
|
|
The address of the second line's first char is 64 regardless of the number of chars/line. Don't forget to clear display content before sending new text etc. |
|
 |
Ttelmah Guest
|
Re: who have sorcecode of LCD in 8 bit mode. Please post me |
Posted: Tue Aug 17, 2004 10:48 am |
|
|
Anonymous wrote: | who have sorcecode of LCD in 8 bit mode. Please post me sourcecode. because my sourcecode is not stable. it have 1 line. sometime it have 2 line. i use 16f877 and lcd 16*2 char. thank you for your help |
There is not really much to it!.
Using PortB for the 8biit data, and three bits defined as 'RW', 'RS', and 'EN' for the control, with portB set to 'fastio':
Code: |
/* These are the values to send to the LCD for special control/setup */
#DEFINE BS 0x08 /* code to backspace */
#DEFINE EMD 0x1E /* program to default entry mode */
#DEFINE CLS 0x12 /* clear screen */
#DEFINE FLINE 0x80 /* move to location */
#DEFINE CON 0x1B /* enable cursor */
#DEFINE COFF 0x1A /* disable cursor */
#DEFINE FSET 0x1F /* set to 2 lines etc. */
#DEFINE DISON 0x1D /* code to switch on display */
#DEFINE DISOFF 0x1C /* code to disable display */
#DEFINE HOME 0x80 /* code to goto home */
#DEFINE LASTTO 0x9F /* Last 'goto' command */
#DEFINE SLINE 0x90 /* code to goto start second line */
#DEFINE CR 0x0C /* carriage return */
#DEFINE LF 0x0A /* line feed */
#DEFINE CLEOL 0x19 /* clear to EOL */
#DEFINE CGW 0x17 /* write to CG RAM */
#DEFINE DW 0x18 /* write to normal RAM */
/* These are the LCD control lines */
#DEFINE EN PIN_C0
#DEFINE RS PIN_C2
#DEFINE RW PIN_C1
void dctl(unsigned int chr)
{
/* this is the code to put one char (chr) to the control port on the LCD */
/* to do this, I need to raise the 'strobe', but leave RS low */
portb=chr;
output_low(RW);
delay_us(1);
output_high(EN);
delay_us(2);
output_low(EN);
}
void ddat(unsigned int chr)
{
/* as above for the data port on the LCD */
portb=chr;
output_low(RW);
output_high(RS);
delay_us(1);
output_high(EN);
delay_us(2);
output_low(EN);
output_low(RS);
}
int checkflag(void) {
int ipval;
SET_TRIS_B(0xFF);
OUTPUT_LOW(RS);
OUTPUT_HIGH(RW);
OUTPUT_HIGH(EN);
delay_us(5);
pval=portb;
OUTPUT_LOW(EN);
OUTPUT_LOW(RW);
SET_TRIS_B(0);
return(ipval & 0x80);
}
|
I have a 'parser', which uses:
Code: |
static int DRAM=true;
static unsigned int dcon = 0x0f;
while (checkflag()) ;
if (chr<0x20)
{
/* here I have a control character */
/* This has the most complex layout, requiring character translation... */
switch (chr) {
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
case 6:
case 7:
ddat(chr); /* send CGRAM display character */
break;
case BS:
/* This is the 'bs' character */
dctl(0x10); /* This shifts left one character */
break;
/* Note that this does not provide the proper 'bs' function. The
input code has to substitute 'bs, space, bs' to get the required
display */
case CLS:
/* CLS */
dctl(1);
break;
case COFF:
/* cursor off */
bit_clear(dcon,1);
bit_clear(dcon,0);
dctl(dcon);
break;
case CON:
bit_set(dcon,1);
bit_set(dcon,0);
dctl(dcon);
break;
case DISOFF:
/* display off */
bit_clear(dcon,2);
dctl(dcon);
break;
case DISON:
bit_set(dcon,2);
dctl(dcon);
break;
case EMD:
/* EMD command (increment, no shift) */
dctl(6);
break;
case FSET:
/* function set */
dctl(0x38);
break;
case CGW:
/* This sets a flag, so that the _next_ address write switches to CGW */
DRAM=FALSE;
break;
case DW:
DRAM=true;
break;
default:
break;
}
}
else if (chr>=HOME && chr<=(LASTTO+1))
{
/* Here I have a 'goto' character */
/* Here the low five bits of the character are the required address */
/* I also have to check whether CGRAM, or DDRAM is required */
if (DRAM)
{
if (chr>=0x90)
dctl(chr+0x30);
else
{
while (true) {
dctl(chr);
while (checkflag()) ;
/* Here I check that address has updated */
SET_TRIS_B(0xFF);
OUTPUT_LOW(RS);
OUTPUT_HIGH(RW);
OUTPUT_HIGH(EN);
delay_us(5);
ipval=portb;
OUTPUT_LOW(EN);
if (ipval == (chr & 0x7F)) {
OUTPUT_LOW(RW);
SET_TRIS_B(0);
break;
}
OUTPUT_LOW(RW);
SET_TRIS_B(0);
}
}
}
else
{
/* Here I want to talk to CGRAM */
dctl(chr-0x40);
}
}
else
{
/* here a normal text character */
ddat(chr);
}
}
}
|
You'll have to count the brackets, since it is cut out of a larger subroutine. The initialisation sequence, is then FSET,FSET,FSET,FSET, DISOFF,DISON,EMD,FLINE.
Best Wishes |
|
 |
treitmey
Joined: 23 Jan 2004 Posts: 1094 Location: Appleton,WI USA
|
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|