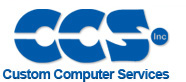 |
 |
View previous topic :: View next topic |
Author |
Message |
rashworth
Joined: 23 Jan 2004 Posts: 4 Location: Silverstone UK
|
ADC on 12F675 |
Posted: Fri Jan 23, 2004 12:58 pm |
|
|
I am having a ferw problems getting the 10-Bit ADC value out of the 12F675. All I want to do is turn two LED's oon depending on the input voltage. The voltage can go between 0-Vdd, and I want 0-1023. The fuses are set using PICSTART ptrogrammer and the program runs, but allways flashes green
Code: | #device PIC12F675
#device ADC=10
//////// Fuses: LP,XT,INTRC,HS,NOWDT,WDT,CPD,NOCPD,PROTECT,NOPROTECT,NOMCLR
//////// Fuses: MCLR,PUT,NOPUT,RC,EC,RC_IO,INTRC_IO,BROWNOUT,NOBROWNOUT
#use delay(clock=4000000)
#define PIN_A0 40
#define PIN_A1 41
#define PIN_A2 42
#define PIN_A3 43
#define PIN_A4 44
#define PIN_A5 45
////////////////////////////////////////////////////////////////// Useful defines
#define FALSE 0
#define TRUE 1
////////////////////////////////////////////////////////////////// ADC
// ADC Functions: SETUP_ADC(), SETUP_ADC_PORTS() (aka SETUP_PORT_A),
// SET_ADC_CHANNEL(), READ_ADC()
// Constants used in SETUP_ADC_PORTS() are: (These may be ORed together)
#define NO_ANALOGS 0
#define ALL_ANALOG 15 //| GP0 GP1 GP2 GP4
#define AN0_ANALOG 1 //| GP0
#define AN1_ANALOG 2 //| GP1
#define AN2_ANALOG 4 //| GP2
#define AN3_ANALOG 8 //| GP4
#define VREF 0x4002
#define VSS_VDD 0x0000
#define VSS_VREF 0x4002 //| Range 0-Vref
// Constants used for SETUP_ADC() are:
#define ADC_OFF 0 // ADC Off
#define ADC_CLOCK_DIV_2 1
#define ADC_CLOCK_DIV_4 0x41
#define ADC_CLOCK_DIV_8 0x11
#define ADC_CLOCK_DIV_16 0x51
#define ADC_CLOCK_DIV_32 0x21
#define ADC_CLOCK_DIV_64 0x61
#define ADC_CLOCK_INTERNAL 0x31 // Internal 2-6us
// Constants used in READ_ADC() are:
#define ADC_START_AND_READ 7 // This is the default if nothing is specified
#define ADC_START_ONLY 1
#define ADC_READ_ONLY 6
#define RED PIN_A4
#define GREEN PIN_A5
//Value to save ADC reading
long Value;
setup_tris_a(0xf);//RA0, RA1, RA2, RA3 as Inputs
//RA4, RA5 as Outputs
setup_adc_ports( AN0_ANALOGUE);
setup_adc(ADC_CLOCK_INTERNAL );
set_adc_channel(0);
void ReadMyAdc()
{
Value=read_adc(adc_read_only);
//If more then 50%
if (Value > 512)//Flash Green
{
output_bit(GREEN,1);
output_bit(RED,0);
delay_ms (250);
output_bit(GREEN,0);
output_bit(RED,0);
delay_ms(250);
}
if (Value < 513)//Flash Red
{
output_bit(GREEN,0);
output_bit(RED,1);
delay_ms (250);
output_bit(GREEN,0);
output_bit(RED,0);
delay_ms(250);
}
}
void main()
{
while(TRUE)
{
ReadMyAdc();
}
}
|
 |
|
 |
Ttelmah Guest
|
Re: ADC on 12F675 |
Posted: Fri Jan 23, 2004 1:09 pm |
|
|
rashworth wrote: | I am having a ferw problems getting the 10-Bit ADC value out of the 12F675. All I want to do is turn two LED's oon depending on the input voltage. The voltage can go between 0-Vdd, and I want 0-1023. The fuses are set using PICSTART ptrogrammer and the program runs, but allways flashes green
Code: | #device PIC12F675
#device ADC=10
//////// Fuses: LP,XT,INTRC,HS,NOWDT,WDT,CPD,NOCPD,PROTECT,NOPROTECT,NOMCLR
//////// Fuses: MCLR,PUT,NOPUT,RC,EC,RC_IO,INTRC_IO,BROWNOUT,NOBROWNOUT
#use delay(clock=4000000)
#define PIN_A0 40
#define PIN_A1 41
#define PIN_A2 42
#define PIN_A3 43
#define PIN_A4 44
#define PIN_A5 45
////////////////////////////////////////////////////////////////// Useful defines
#define FALSE 0
#define TRUE 1
////////////////////////////////////////////////////////////////// ADC
// ADC Functions: SETUP_ADC(), SETUP_ADC_PORTS() (aka SETUP_PORT_A),
// SET_ADC_CHANNEL(), READ_ADC()
// Constants used in SETUP_ADC_PORTS() are: (These may be ORed together)
#define NO_ANALOGS 0
#define ALL_ANALOG 15 //| GP0 GP1 GP2 GP4
#define AN0_ANALOG 1 //| GP0
#define AN1_ANALOG 2 //| GP1
#define AN2_ANALOG 4 //| GP2
#define AN3_ANALOG 8 //| GP4
#define VREF 0x4002
#define VSS_VDD 0x0000
#define VSS_VREF 0x4002 //| Range 0-Vref
// Constants used for SETUP_ADC() are:
#define ADC_OFF 0 // ADC Off
#define ADC_CLOCK_DIV_2 1
#define ADC_CLOCK_DIV_4 0x41
#define ADC_CLOCK_DIV_8 0x11
#define ADC_CLOCK_DIV_16 0x51
#define ADC_CLOCK_DIV_32 0x21
#define ADC_CLOCK_DIV_64 0x61
#define ADC_CLOCK_INTERNAL 0x31 // Internal 2-6us
// Constants used in READ_ADC() are:
#define ADC_START_AND_READ 7 // This is the default if nothing is specified
#define ADC_START_ONLY 1
#define ADC_READ_ONLY 6
#define RED PIN_A4
#define GREEN PIN_A5
//Value to save ADC reading
long Value;
setup_tris_a(0xf);//RA0, RA1, RA2, RA3 as Inputs
//RA4, RA5 as Outputs
setup_adc_ports( AN0_ANALOGUE);
setup_adc(ADC_CLOCK_INTERNAL );
set_adc_channel(0);
void ReadMyAdc()
{
Value=read_adc(adc_read_only);
//If more then 50%
if (Value > 512)//Flash Green
{
output_bit(GREEN,1);
output_bit(RED,0);
delay_ms (250);
output_bit(GREEN,0);
output_bit(RED,0);
delay_ms(250);
}
if (Value < 513)//Flash Red
{
output_bit(GREEN,0);
output_bit(RED,1);
delay_ms (250);
output_bit(GREEN,0);
output_bit(RED,0);
delay_ms(250);
}
}
void main()
{
while(TRUE)
{
ReadMyAdc();
}
}
|
 |
You are not initialising the ADC.
The instructions:
setup_tris_a(0xf);//RA0, RA1, RA2, RA3 as Inputs
//RA4, RA5 as Outputs
setup_adc_ports( AN0_ANALOGUE);
setup_adc(ADC_CLOCK_INTERNAL );
set_adc_channel(0);
Need to be _in_ the code somewhere. You have them as 'definitions' (effectively they will be working as function prototypes), but you never actually call them. Insert these at the front of your 'main'.
Best Wishes |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|